As I said earlier, the page can have some functions like
rating system, commenting system etc. These functionalities can be implemented
in MasterPage itself. The PageID(or a ArticleID) in @Page Directive will help us
identify the article for which the comment and rating should be stored in
database. We can also implement these functionalities through a user control and
can include it in the master page. The title that is set on the Title attribute
can be assigned to lblPageHead in MasterPage.
Refer the below Codebehind file of the master page for
clear understanding.
public partial class MainMaster :
System.Web.UI.MasterPage
{
private string _pageID;
public string PageID
{
get
{
return _pageID;
}
set
{
_pageID = value;
}
}
protected void Page_Load(object sender, EventArgs
e)
{
this.lblPageHead.Text =this.Page.Title;
}
}
The PageID value can be used to store the comments,
ratings, etc from Master Page.
Dynamic ASPX Page Generation
We will build a page that accepts Title, Meta Keyword,
Meta Description and Content from the user and replace it with the placeholders
that are in Template.aspx page and save it as a separate aspx page. Refer the
below diagram.
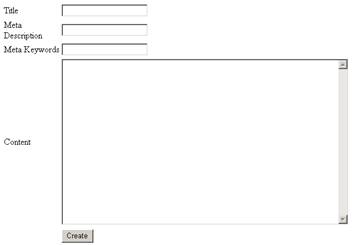
The dynamic page creation cod will be,
protected void btnCreate_Click(object sender, EventArgs
e)
{
string root = Server.MapPath("~");
//Read the Template file
string Template = root +
"\\PageTemplate.temp";
StringBuilder line = new StringBuilder();
using (StreamReader rwOpenTemplate = new
StreamReader(Template))
{
while (!rwOpenTemplate.EndOfStream)
{
line.Append(rwOpenTemplate.ReadToEnd());
}
}
int ID = 0;
string SaveFilePath = "";
string SaveFileName = "";
Random ran = new Random();
ID = ran.Next();
//Page Name Creator with only URL allowed
character
string Title = ID.ToString() + "-" + StripURLNotAllowedChars(txtTitle.Text);
SaveFileName = "\\"+ Title + ".aspx";
SaveFilePath = root + "\\Pages\\" +
SaveFileName;
FileStream fsSave =
File.Create(SaveFilePath);
if (line != null)
{ //Replace the page
content
line.Replace("[Title]",
txtTitle.Text.Replace("<", "<").Replace(">",
">").Replace('"', ' ').Replace('"', ' '));
line.Replace("[PageContent]",
txtContent.Text);
line.Replace("[MetaDes]",
txtDes.Text.Replace('"', ' ').Replace('"', ' ').Replace('<',
'-').Replace('>', '-') );
line.Replace("[key]",
txtKey.Text.Replace('"', ' ').Replace('"', ' ').Replace('<',
'-').Replace('>', '-'));
line.Replace("[ID]",
ID.ToString());
StreamWriter sw = null;
try
{//write content
sw = new StreamWriter(fsSave);
sw.Write(line);
}
catch (Exception ex)
{
lblMessage.Text = ex.Message;
}
finally
{
sw.Close();
}
}
}
private string StripURLNotAllowedChars(string htmlString)
{
string pattern = @"\s|\#|\$|\&|\||\!|\@|\%|\^|\*|\<\|\>|\\|\/|\+|\-|\=";
return Regex.Replace(htmlString, pattern, "-");
}
In the above code, we are reading the template aspx
markup from a file to a StringBuilder object “line”. You can choose your own way
of handling this Template page i.e. you can also store in a table and retrieve
it when necessary.
From the Title of the page that is typed in by the user
we are creating the Page name. The Title that is inputted by user can have space
and URL not allowed characters which should be replaced with a URL allowed
characters like “-“. Thus, the page name itself will describe the content which
is more search engine friendly. We can also append an ID which will make the
page name unique and the same number can be used to identify the article/page in
database to store comments, ratings, etc.
Finally, all the placeholders should be replaced with
the user contents and values.
For example, [Title] with the text of “txtTitle” TextBox
that is typed in by the user.
This approach can be used as an example and we can
refine it further to fit to our need and also we can improve it further for
better performance. Download the code to understand it better.
Deployment
If you see the Template.aspx page we are referring the
codebehind class through CodeFile attribute in @Page directive which is fine when we are actually developing.
This needs to be changed when we deploy so that the assembly is referred in the @Page directive.
We can remove CodeFile attribute and refer the assembly through Inherits attribute.
To deploy the application, we can create a single file assembly for the
project and set the Inherits attribute to the assembly type. To know more about creating single file assembly,
refer my article Web Deployment Plugin for Visual Studio.
Refer the below sample aspx page that is created dynamically for better understanding.
<%@ Page Language="C#"
MasterPageFile="MainMaster.master" AutoEventWireup="true"
Inherits="CommonCodeBehind, Sample_deploy" CodeID="3"
Title="Connecting to Excel sheet using Generic Data Access in ADO.Net 2.0"
MetaDescription="Connecting to Excel sheet in C#, ADO.Net 2.0 and usage of
Generic Data Access in ADO.Net 2.0" %>
<asp:Content ID="Content1"
ContentPlaceHolderID="ContentPlaceHolder1" Runat="Server">
<P class=MsoNormal style="MARGIN: 0in 0in 0pt">The
below code can be used to fetch data from excel sheet using ADO.Net 2.0.
</P>
<P class=MsoNormal style="MARGIN: 0in 0in 0pt">I have
used Generic data access code to achieve this.</P>
<P class=MsoNormal style="MARGIN: 0in 0in
0pt"><?xml:namespace prefix = o ns =
"urn:schemas-microsoft-com:office:office"
/><o:p> </o:p></P>
<P class=MsoNormal style="MARGIN: 0in 0in
0pt"><STRONG><U>Connect to
Excel</U></STRONG></P>
<P class=MsoNormal style="MARGIN: 0in 0in
0pt"><FONT color=#a52a2a>public DataTable
FillDtFromExcel()</FONT></P>
<P class=MsoNormal style="MARGIN: 0in 0in
0pt"><FONT color=#a52a2a><SPAN style="mso-spacerun:
yes">
</SPAN>connection.ConnectionString =
connectionString;</FONT></P>
<P class=MsoNormal style="MARGIN: 0in 0in
0pt"><SPAN style="mso-tab-count: 1"><FONT
color=#a52a2a>
</FONT></SPAN></P>
<P class=MsoNormal style="MARGIN: 0in 0in
0pt"><FONT color=#a52a2a><SPAN style="mso-tab-count:
1">
</SPAN><SPAN style="mso-spacerun:
yes"> </SPAN>DbCommand selectCommand =
factory.CreateCommand();</FONT></P>
<P class=MsoNormal style="MARGIN: 0in 0in
0pt"><FONT color=#a52a2a><SPAN style="mso-spacerun:
yes">
</SPAN>selectCommand.CommandText = query;</FONT></P>
<P class=MsoNormal style="MARGIN: 0in 0in
0pt"><FONT color=#a52a2a><SPAN style="mso-tab-count:
1">
</SPAN>selectCommand.Connection = connection;</FONT></P>
<P class=MsoNormal style="MARGIN: 0in 0in
0pt"><FONT color=#a52a2a><SPAN style="mso-spacerun:
yes">
</SPAN>}</FONT></P>
<P class=MsoNormal style="MARGIN: 0in 0in
0pt"><o:p> </o:p></P>
<P class=MsoNormal style="MARGIN: 0in 0in
0pt">Replace the Excel file name in the connection string with path to use
the above code! The worksheet name should be given inside the [] brackets with a
$ symbol concatenated in the query([AllEmail$]).</P>
</asp:Content>
You can consider some of the free rich textbox controls
to input the page contents.
|