Using
ADRotator Control in ASP.Net-Part 1
Using
ADRotator Control in ASP.Net-Part 2
The main disadvantage of using
AdRotator control is, it will change the advertisement only when there is a postback in the webpage.
Moving forward, we will see how to
rotate or change advertisements without using AdRotator control and postback by
using jquery library. For easy understanding(also to efficiently manage), we will store the ad information in
SQL express database and will use JSON format to return the ads from database.
Steps
1. With Visual Studio 2008, Create a sample Asp.Net website project.
2. Choose a language in the Language Select box. I have selected C# as my language of choice. Name
your website as per the need.
3. Get the latest jQuery library from official jQuery.com site and integrate into
your project. The following Faq’s will demonstrate how to integrate jQuery library into your
project.
What
is jQuery? How to use it in ASP.Net Pages?
How
to enable jQuery intellisense in Visual Studio 2008?
How
to use jQuery intellisense in an external javascript file?
4. Create a new SQL express database(free database edition packed with visual studio) inside App_Data folder
and create a table called Ads with necessary columns using “Server
Explorer”.
Refer the below figure,
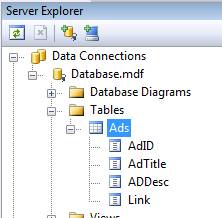
5. In order to fetch the ads from database, we will create an
HttpHandler which can read from the database and return the advertisements in
JSON format. To create an HttpHandler, right click the project in your solution
explorer and click “Add New Item”. Select “Generic Handler”. Rename it to GetAds.ashx and click OK.
In ProcessRequest() event, we can read the ads from database and
return it as a JSON string.
Refer the
below code,
<%@ WebHandler Language="C#"
Class="GetAds" %>
using System;
using System.Web;
using System.Data;
using System.Text;
using System.Data.SqlClient;
using System.Configuration;
using
System.Web.Script.Serialization;
public class GetAds : IHttpHandler
{
public void ProcessRequest
(HttpContext context) {
string query = "SELECT * FROM
[Ads]";
DataTable dt =
GetDt(query);
StringBuilder strArticles = new
StringBuilder();
if (dt != null)
{
strArticles.Append("[");
for (int i = 0; i <
dt.Rows.Count; i++)
{
strArticles.Append("{");
strArticles.Append("\"AdID\":\"" + dt.Rows[i]["AdID"].ToString() +
"\",");
strArticles.Append("\"AdTitle\":\"" + dt.Rows[i]["AdTitle"].ToString() +
"\",");
strArticles.Append("\"ADDesc\":\"" + dt.Rows[i]["ADDesc"].ToString() +
"\",");
strArticles.Append("\"Link\":\"" + dt.Rows[i]["Link"].ToString() +
"\"");
if (i != dt.Rows.Count -
1)
{
strArticles.Append("},");
}
}
}
strArticles.Append("}");
strArticles.Append("]");
context.Response.ContentType =
"application/json";
context.Response.ContentEncoding =
Encoding.UTF8;
context.Response.Write(strArticles.ToString());
context.Response.End();
}
public bool IsReusable {
get {
return false;
}
}
public DataTable GetDt(string
query)
{
SqlConnection con = new
SqlConnection(ConfigurationManager.ConnectionStrings["Sql"].ConnectionString);
SqlDataAdapter ada = new
SqlDataAdapter(query, con);
DataTable dt = new
DataTable();
ada.Fill(dt);
return dt;
}
}
Web.Config
<connectionStrings>
<add name="Sql"
connectionString="Data
Source=.\SQLEXPRESS;AttachDbFilename=|DataDirectory|\Database.mdf;Integrated
Security=True;User Instance=True"
providerName="System.Data.SqlClient"/>
</connectionStrings>
6. Now, we have created our HttpHandler which will return the ads when
called. In order to display the ad in the webpage, we will define a html container(basically a div tag) in
which we can rotate the text ads. In other words, you can place this div tag wherever you want to display the ads in your page.
Open your Default.aspx, declare a div tag. Refer below,
<div id="dvADContainer">
<div
id="dvAD"></div>
</div>
7. Next, we will consume our HttpHandler from jquery and get the ads in
JSON format.
For this we
will use the getJSON() ajax method available in jQuery. To rotate the ads in
equal interval, we will use the setInterval() method available in javascript.
Refer the below code,
|