<style>
/*CollapsiblePanel*/
.ContainerPanel
{
width:400px;
border:1px;
border-color:#1052a0;
border-style:double double double double;
}
.collapsePanelHeader
{
width:400px;
height:30px;
background-image: url(images/bg-menu-main.png);
background-repeat:repeat-x;
color:#FFF;
font-weight:bold;
}
.HeaderContent
{
float:left;
padding-left:5px;
}
.Content
{
}
.ArrowExpand
{
background-image: url(images/expand_blue.jpg);
width:13px;
height:13px;
float:right;
margin-top:7px;
margin-right:5px;
}
.ArrowExpand:hover
{
cursor:hand;
}
.ArrowClose
{
background-image: url(images/collapse_blue.jpg);
width:13px;
height:13px;
float:right;
margin-top:7px;
margin-right:5px;
}
.ArrowClose:hover
{
cursor:hand;
}
</style>
Next, we will implement the
expand/collapse functionality using jquery.
To do this, we will use the toggle
function in jquery library to add the expand collapse functionality to the div.
Refer the below code.
<script src="_scripts/jquery-1.2.6.js"
type="text/javascript"></script>
<script language="javascript">
$(document).ready(function() {
$("DIV.ContainerPanel >
DIV.collapsePanelHeader > DIV.ArrowExpand").toggle(
function() {
$(this).parent().next("div.Content").show("slow");
$(this).attr("class",
"ArrowClose");
},
function() {
$(this).parent().next("div.Content").hide("slow");
$(this).attr("class",
"ArrowExpand");
});
});
</script>
In the above code, i have made the element selection using
tag name and css class i.e.
$("DIV.ContainerPanel > DIV.collapsePanelHeader >
DIV.ArrowExpand”)
By doing the above, i have prevented
the selections based on id of the DIV tag because we can use the same code if we
have multiple collapsible panel in a page. The toggle function will be
attached to all the matched element found for the selector
expression in that page and hence the above code will work fine even if we
have more than one collapsible panel in a single page. Download the source
attached in "Downloads" section and see it in action. Refer the below figure to
understand how multiple collapsible panel in a page will look like,
Multiple Collapsible panels in a
page
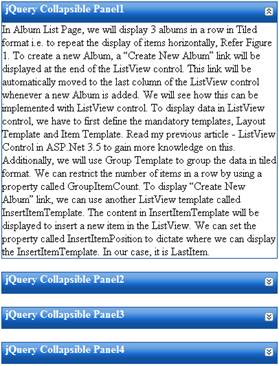
You can use below HTML to construct the above multiple collapsible
panels.
<div id="ContainerPanel" class="ContainerPanel">
<div id="header" class="collapsePanelHeader">
<div id="dvHeaderText"
class="HeaderContent">jQuery Collapsible Panel1</div>
<div id="dvArrow"
class="ArrowExpand"></div>
</div>
<div id="dvContent" class="Content"
style="display:none">
/*Content*/
</div>
</div>
<br />
<div id="Div1" class="ContainerPanel">
<div id="Div2" class="collapsePanelHeader">
<div id="Div11" class="HeaderContent">jQuery
Collapsible Panel2</div>
<div id="Div3"
class="ArrowExpand"></div>
</div>
<div class="Content"
style="display:none">
/*Content*/
</div>
</div>
<br />
<div id="Div4" class="ContainerPanel">
<div id="Div5" class="collapsePanelHeader">
<div id="Div12"
class="HeaderContent">jQuery Collapsible Panel3</div>
<div id="Div6"
class="ArrowExpand"></div>
</div>
<div class="Content"
style="display:none">
/*Content*/
</div>
</div>
<br />
<div id="Div7" class="ContainerPanel">
<div id="Div8" class="collapsePanelHeader">
<div id="Div13"
class="HeaderContent">jQuery Collapsible Panel4</div>
<div id="Div9"
class="ArrowExpand"></div>
</div>
<div id="Div10" class="Content"
style="display:none">
/*Content*/
</div>
</div>
Execute the page to see it in action.
|