I have only hard coded for 2 states,
you can loop through the result set from database and give back the data in JSON
format.
Calling HttpHanlder from jQuery and
building RadioButtonList control
Read the following FAQ’s to integrate
and use jQuery in Visual Studio 2008,
What
is jQuery? How to use it in ASP.Net Pages?
How
to enable jQuery intellisense in Visual Studio 2008?
How
to use jQuery intellisense in an external javascript file?
We will declare an onchange event for
the parent DropDownList which will make the AJAX call to the server to fetch the
JSON data.
Refer the below code,
<script src="_scripts/
jquery-1.3.2.min.js" type="text/javascript"></script>
<script
language="javascript">
$(document).ready(function()
{
$("#ddlStates").change(function() {
$('#rdbList').html("");
var StateID =
$("#ddlStates > option[selected]").attr("value");
if (StateID != 0)
{
$.getJSON('LoadCities.ashx?StateID=' + StateID, function(cities) {
var i =
0;
$.each(cities,
function() {
var rdb =
"<input id=RadioButton" + i + " onclick=RecordCheck(this) type=radio
name=City value=" + this['ID'] + " /><label for=RadioButton" + i + ">"
+ this['City'] + "</label>";
$('#rdbList').append(rdb);
i++;
});
});
}
});
});
function RecordCheck(rdb)
{
$('#hfCity').val(rdb.value);
}
</script>
The getJSON method will be called for
every state selection except for the value of “select” in parent DropDownList.
When the JSON result is returned it will call the call-back
function(2nd argument of getJSON method) which will populate the
RadioButtonList control inside the DIV tag.
The function RecordCheck() will be
called once the user selects any city and the corresponding value will get
recorded in the hidden field.
Accessing the selected
Value
In serverside, we can get the city
selected from the hidden field.
Refer below,
protected void btnSave_Click(object
sender, EventArgs e)
{
Response.Write(hfCity.Value);
}
Execute the page and see it in
action.
One of the problems in this approach
is, once we click save, the radio buttons we have populated through jQuery will
not persist and will get cleared off from the form.
Refer the below figure,
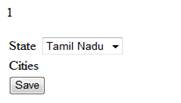
This is because; asp.net will maintain
the page control’s state in viewstate which it uses to rebuild the control on
every postback. Since, we are building the control in client side script asp.net
will not have any information to render it back to the browser.
If you would like to retain the radio
buttons and selection then we can include an ASP.Net RadioButtonList and can
bind it in the save event. The city selection can be done again based on the
value in HiddenField.
The final code will look
like,
ASPX
<table>
<tr>
<td>State</td>
<td>
<asp:DropDownList
ID="ddlStates" runat="server">
<asp:ListItem
Value="0">Select</asp:ListItem>
<asp:ListItem
Value="1">Tamil Nadu</asp:ListItem>
<asp:ListItem
Value="2">Karnataka</asp:ListItem>
</asp:DropDownList>
</td>
</tr>
<tr>
<td>Cities</td>
<td>
<DIV
id="rdbList">
</DIV>
<asp:HiddenField
ID="hfCity" runat="server"></asp:HiddenField>
<asp:RadioButtonList
ID="rdbCities" runat="server">
</asp:RadioButtonList>
</td>
</tr>
<tr>
<td colspan="2">
<asp:Button ID="btnSave"
runat="server" Text="Save" onclick="btnSave_Click" />
</td>
</tr>
</table>
CodeBehind
protected void btnSave_Click(object
sender, EventArgs e)
{
if (rdbCities.Items.Count > 0
&& hfCity.Value == "")
{
Response.Write(rdbCities.SelectedValue);
}
else
{
Response.Write(hfCity.Value);
string StateId =
ddlStates.SelectedValue;
LoadCities(StateId);
if (hfCity.Value !=
"")
{
rdbCities.Items.FindByValue(hfCity.Value).Selected = true;
hfCity.Value =
"";
}
}
}
public void LoadCities(string
StateId)
{
if (StateId == "1")
{
rdbCities.Items.Clear();
rdbCities.Items.Add(new
ListItem("Chennai", "1"));
rdbCities.Items.Add(new
ListItem("Coimbatore", "2"));
}
else if (StateId ==
"2")
{
rdbCities.Items.Clear();
rdbCities.Items.Add(new
ListItem("Bangalore", "1"));
rdbCities.Items.Add(new
ListItem("Belgaum", "2"));
}
}
|