
It will be good and user friendly, if we provide a button
next to the textbox which will pop up a calendar for the user to select a date,
a datepicker control. This prevents the need to make the user type in a
particular format and also will give a better user experience. Well, this can be
implemented in various ways. This article will help us to build this control
ourselves and also some of the other options available. Moving forward, this
article will help us to create DatePicker control in 3 ways,
Ø Using
ASP.Net Calendar control.
Ø Using
Calendar Extender control in AJAX Control Toolkit.
Ø Using
jQuery DatePicker Plug-in.
Using ASP.Net Calendar control
1) Drag a TextBox to the WebForm and
name it as 'txtDate'.
2) You can either use button control or
an IMG to open the calendar.
Now write a JavaScript function to open
the popup that is containing the Calender control.
function PopupPicker(ctl)
{
var PopupWindow=null;
PopupWindow=window.open('DatePicker.aspx?ctl='+ctl,'','width=250,height=250');
PopupWindow.focus();
}
Pass the textbox control name as a
query string (here ctl) to the popup, so that the pop up window can populate the
textbox control in the parent window with the date selected by you. I have used
image button to open the Calendar. Refer the below code,
<img src="_images/images.jpg"
style="cursor:hand;" onclick="PopupPicker('txtDate')" />
Since, we are passing the textbox id as
a querystring we can reuse this control in multiple places in our project.
Means, just pass the textbox id where you want to populate the date in the
onclick function call.
Building DatePicker.aspx
1) Drag a Calendar Control into the
webform.
2) Get the TextBox Control Name that is
coming as query string.
3) Fill the Parent form's textbox with
the selected date by using the following JavaScript function.
function SetDate(dateValue,ctl)
{
thisForm =
window.opener.document.forms[0].elements[ctl].value= dateValue;
self.close();
}
Now in clnDatePicker_DayRender Event,
write the follow code to call the javascript function 'SetDate'.
protected void Calendar1_DayRender(object
sender, DayRenderEventArgs e)
{
string ctrl =
Request.QueryString["ctl"];
HyperLink hpl = new
HyperLink();
hpl.Text =
((LiteralControl)e.Cell.Controls[0]).Text;
hpl.NavigateUrl = "javascript:SetDate('" +
e.Day.Date.ToShortDateString() + "','"+ctrl+"');";
e.Cell.Controls.Clear();
e.Cell.Controls.Add(hpl);
}
By default, all the day links rendered
by the calendar server control will generate a postback to the server. What we
do here is instead of postback, we will call our custom SetDate() JavaScript
function.
To do this, we will clear the table
cell that contains the day link and replace with a HyperLink control with our
javascript function attached to that. Refer the above code.
Execute the page and see it in action.
Using Calendar Extender control in AJAX
Control Toolkit
The next option to provide a datepicker
feature in asp.net is using the Calendar extender control from Ajax control
toolkit.
How to construct this?
To use this feature, you need to
download Ajax control toolkit from here.
Read my article Ajax
Control Toolkit – Introduction to integrate and know more about
this toolkit.
I assume you have toolkit already
installed and integrated in your visual studio.
1. Drag a textbox control.
2. Drag a CalenderExtender control.
3. Set the textbox ID in the TargetControlID property of
CalenderExtender control.
<cc1:ToolkitScriptManager
ID="ToolkitScriptManager1" runat="server">
</cc1:ToolkitScriptManager>
<asp:TextBox ID="txtDate"
runat="server"></asp:TextBox>
<cc1:CalendarExtender
ID="CalendarExtender1" TargetControlID="txtDate" runat="server">
</cc1:CalendarExtender>
Execute the page. You can see the
datepicker control in action similar to below figure.
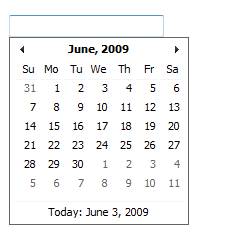
The drawbacks of this approach
are,
1. This technique has a dependency on ASP.Net AJAX framework and hence
it requires the framework to be installed on the production server.
2. Your page will be heavy due to the ASP.NET Ajax client-side framework
scripts.
Next, we will see how we can provide
datepicker feature using jQuery.
|