Difference between Custom Control and
User Control
A custom control is one that works
similar to existing asp.net server control which has separate assembly and
lifecycle of an asp.net server control. Since, it produces a dll; it can re-used
in multiple projects like any other server control. A usercontrol is one which
can be created using the existing server controls to satisfy a requirement that
can be reused in a project. A user control can be compared with the include
files in classic asp application and it works quite similar to a normal aspx
page.
For example, user controls in asp.net
1.x are commonly used for providing common header and footer for a website.
Another common use is for providing login box in every pages of the
website.
Moving forward, we will see more about
user controls, dynamically loading user controls and some advance usages with
user controls. To understand better, we will build a RSS reader usercontrol that
can be used across the project.
Steps
1. Open Visual Studio 2005/2008.
2. Click File > New > Website.
3. Select ASP.Net Website. Also, select the language of your choice. I
have selected C#. Name your website accordingly.
4. To add a new usercontrol, right click your project in the solution
explorer > Add new item.
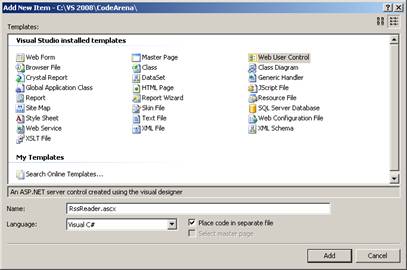
5. We will use DataList control to populate the RSS feed and display it
to the users. So, Drag a DataList control from Data tab of Visual Studio into
our user control. Drag an ASP.Net label control to display error
message.
The final code will look
like,
<%@ Control Language="C#"
AutoEventWireup="true" CodeFile="RssReader.ascx.cs" Inherits="RssReader"
%>
<asp:DataList ID="dlRSS" runat="server"
Width="100%">
<ItemTemplate>
<div
class="RSSTitle"><asp:HyperLink ID="TitleLink" runat="server" Text='<%#
Eval("title") %>' NavigateUrl='<%# Eval("link")
%>'/></div>
<div
class="RSSSubtitle"><asp:Label ID="SubtitleLabel" runat="server"
Text='<%# Eval("description") %>' /></div>
<div
class="RSSInfo">
posted on
<asp:Label ID="DateRSSedLabel" runat="server" Text='<%# Eval("pubDate",
"{0:d} @ {0:t}") %>' />
</div>
</ItemTemplate>
</asp:DataList>
<asp:Label ID="lblMessage"
runat="server" Text="Label"></asp:Label>
6. In the code behind file of the user control, we will read the RSS
content and bind it to the DataList using XmlTextReader and DataSet object. I
have used www.asp.net rss to bind the
DataList.
public partial class RssReader :
System.Web.UI.UserControl
{
protected void Page_Load(object
sender, EventArgs e)
{
XmlTextReader reader =
null;
try
{
reader = new
XmlTextReader("http://www.asp.net/community/articles/rss.ashx");
DataSet ds = new
DataSet();
ds.ReadXml(reader);
dlRSS.DataSource =
ds.Tables["item"];
dlRSS.DataBind();
}
catch (Exception ex)
{
lblMessage.Text =
ex.Message;
}
finally
{
reader.Close();
}
}
}
The lblMessage is ASP.Net label control
to display error message.
7. To use this user control in an aspx page, drag the ascx file from the
solution explorer to the aspx page. This will add a Register directive (just
below the Page Directive) and control markup in the aspx page. Refer the below
code,
<%@ Register Src="RssReader.ascx"
TagName="RssReader" TagPrefix="uc1" %>
<uc1:RssReader ID="RssReader1"
runat="server" />
Execute the page and see it in action.
In the same way, you can add the user control to the other pages as
well.
If you see our usercontrol, we have
hard coded the RSS URL to the asp.net site. Consider, if we want to make this as
a configurable and more generic i.e. it will be good if we expose a property in
the usercontrol that accepts any RSS URL and fetches the content from that URL.
Next section will help us to do this.
|