Sort by clicking up and down arrow image
It will be better and user friendly if we can display up
and down arrow image to indicate the sort direction instead of making the
ListView header clickable as demonstrated in the previous section. Something
like below,
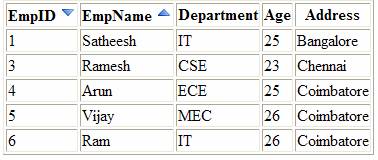
To do this, we need to replace the LinkButton to
ImageButton and provide the header text separately. To toggle between up and
down arrow image, we will use the onsorting event raised by the ListView
control. Again, similar to previous approach, we need to set CommandArgument
property of the ImageButton to the database column name and CommandName to
“Sort” in order to fire the onsorting event. The below code does that,
ASPX
<asp:ListView ID="lvEmployees" runat="server"
DataSourceID="SqlDataSource1" ItemPlaceholderID="itemPlaceholder"
onsorting="lvEmployees_Sorting">
<LayoutTemplate>
<table ID="itemPlaceholderContainer" border="1">
<tr id="Tr1">
<th id="Th1">
EmpID
<asp:ImageButton ID="imEmpID"
CommandArgument="EMPID" CommandName="Sort" ImageUrl="~/img/asc.png"
runat="server" />
</th>
<th id="Th2">
EmpName
<asp:ImageButton ID="imEmpName"
CommandArgument="EMPNAME" CommandName="Sort" ImageUrl="~/img/asc.png"
runat="server" />
</th>
<th id="Th3">
Department</th>
<th id="Th4">
Age</th>
<th id="Th5">
Address</th>
</tr>
<tr ID="itemPlaceholder" runat="server">
</tr>
</table>
</LayoutTemplate>
<ItemTemplate>
<tr>
<td>
<asp:Label ID="EmpIDLabel" runat="server"
Text='<%# Eval("EmpID") %>' />
</td>
<td>
<asp:Label ID="EmpNameLabel" runat="server"
Text='<%# Eval("EmpName") %>' />
</td>
<td>
<asp:Label ID="DepartmentLabel"
runat="server" Text='<%# Eval("Department") %>' />
</td>
<td>
<asp:Label ID="AgeLabel" runat="server"
Text='<%# Eval("Age") %>' />
</td>
<td>
<asp:Label ID="AddressLabel" runat="server"
Text='<%# Eval("Address") %>' />
</td>
</tr>
</ItemTemplate>
</asp:ListView>
<asp:SqlDataSource ID="SqlDataSource1"
runat="server"
ConnectionString="<%$
ConnectionStrings:DatabaseConnectionString %>"
SelectCommand="SELECT * FROM
[Employee]"></asp:SqlDataSource>
CodeBehind
protected void lvEmployees_Sorting(object sender,
ListViewSortEventArgs e)
{
ImageButton imEmpID =
lvEmployees.FindControl("imEmpID") as ImageButton;
ImageButton imEmpName =
lvEmployees.FindControl("imEmpName") as ImageButton;
string DefaultSortIMG = "~/img/asc.png";
string imgUrl = "~/img/desc.png";
if (e.SortDirection ==
SortDirection.Descending)
{
imgUrl = DefaultSortIMG;
}
switch (e.SortExpression)
{
case "EMPID":
if (imEmpName != null)
imEmpName.ImageUrl =
DefaultSortIMG;
if (imEmpID != null)
imEmpID.ImageUrl = imgUrl;
break;
case "EMPNAME":
if (imEmpID != null)
imEmpID.ImageUrl = DefaultSortIMG;
if (imEmpName != null)
imEmpName.ImageUrl = imgUrl;
break;
}
}
In the above code, I am swapping the images based on the
value of e.SortDirection property in onsorting event.
Download the source code attached in this article and
see it in action.
|