Moving forward, let’s understand how to deal with this
challenge and implement a working sorting feature on the ListView control when
manually binding the data from codebehind file.
Similar to my previous article, I will demonstrate
sorting in two different ways,
1. Sorting ListView by clicking
the header link
2. Sorting ListView by clicking up
and down arrow image
I will also use the same database table and ListView
layout which we used in the previous part in this article too.
To understand this article, I assume you have created a
new Asp.Net project using C# as language in your visual studio 2008.
Sorting ListView by clicking the header link
Drag a ListView control from the data tab of your visual
studio 2008. Configure the LayoutTemplate and ItemTemplate of ListView control
to display the data. Like I said earlier, I will use tabular format in this
article. Read the article ListView
Control in ASP.Net 3.5 if you are new to ListView control. To make a column
sortable, we need to define the header as a LinkButton by setting its
CommandArgument property to the database column name and CommandName to “Sort”.
When this settings, it will fire onsorting event of ListView control whenever
the header text is clicked. Refer the below code,
ASPX
<asp:ListView ID="lvEmployee" runat="server"
DataKeyNames="EmpID" onsorting="lvEmployee_Sorting">
<LayoutTemplate>
<table ID="itemPlaceholderContainer"
border="1">
<tr id="Tr2">
<th id="Th1">
<asp:LinkButton ID="lbEmpID"
CommandArgument="EMPID" CommandName="Sort" Text="EmpID" runat="server" />
</th>
<th
id="Th2">
<asp:LinkButton ID="lbEmpName"
CommandArgument="EMPNAME" CommandName="Sort" Text="EmpName" runat="server"
/>
</th>
<th id="Th3">
Department</th>
<th id="Th4">
Age</th>
<th id="Th5">
Address</th>
</tr>
<tr ID="itemPlaceholder" runat="server">
</tr>
</table>
</LayoutTemplate>
<ItemTemplate>
<tr>
<td>
<asp:Label ID="EmpIDLabel" runat="server"
Text='<%# Eval("EmpID") %>' />
</td>
<td>
<asp:Label ID="EmpNameLabel" runat="server"
Text='<%# Eval("EmpName") %>' />
</td>
<td>
<asp:Label ID="DepartmentLabel" runat="server"
Text='<%# Eval("Department") %>'
/>
</td>
<td>
<asp:Label ID="AgeLabel" runat="server"
Text='<%# Eval("Age") %>' />
</td>
<td>
<asp:Label ID="AddressLabel" runat="server"
Text='<%# Eval("Address") %>' />
</td>
</tr>
</ItemTemplate>
</asp:ListView>
CodeBehind
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
BindLV("");
}
public DataTable GetEmployee(string query)
{
SqlConnection con = new
SqlConnection(ConfigurationManager.ConnectionStrings["DatabaseConnectionString"].ConnectionString);
SqlDataAdapter ada = new SqlDataAdapter(query,
con);
DataTable dtEmp = new DataTable();
ada.Fill(dtEmp);
return dtEmp;
}
private void BindLV(string SortExpression)
{
lvEmployee.DataSource = GetEmployee("Select * from
Employee" + SortExpression);
lvEmployee.DataBind();
}
protected void lvEmployee_Sorting(object sender,
ListViewSortEventArgs e)
{
LinkButton imEmpID =
lvEmployee.FindControl("imEmpID") as LinkButton;
LinkButton imEmpName =
lvEmployee.FindControl("imEmpName") as LinkButton;
string SortDirection = "ASC";
if (ViewState["SortExpression"] != null)
{
if (ViewState["SortExpression"].ToString() ==
e.SortExpression)
{
ViewState["SortExpression"] = null;
SortDirection = "DESC";
}
else
{
ViewState["SortExpression"] =
e.SortExpression;
}
}
else
{
ViewState["SortExpression"] =
e.SortExpression;
}
BindLV(" order by " + e.SortExpression + " " +
SortDirection);
}
In the above code, I have made EmpID and EmpName columns
to be sortable in the ListView control. Since e.SortDirection property will not
remember the correct sort direction, I have used ViewState to hold the last sort
expression to manipulate the sort direction in onsorting event. That’s it!
Execute the application and see it in action. Refer the below screen shot for
the output.
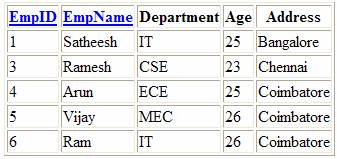
|