Below are the set of validation control
that are packed with the ASP.Net.
Ø RequiredFieldValidator control
Ø RegularExpressionValidator control
Ø RangeValidator control
Ø CompareValidator control
Ø CustomValidator control
Ø ValidationSummary control
How these Validator control
work?
To make this validation control to
work, asp.net will render a javascript file that contains the validation scripts
to client side when the page is rendered. From ASP.Net 2.0, these inbuilt
validation script will be rendered using WebResource.axd handler due to some
security reasons. In 1.x days, these will be rendered as a javascript file
"WebUIValidation.js" and it will be referred in the page using the script
tag.
Let us see about these validation
controls in coming sections of this article.
RequiredFieldValidator control
As the name suggests, this control is
used to do the mandatory check of a field in ASP.Net. For example, in an input
entry form to make a name as mandatory we can use this control. Using this
control is pretty simple.
Refer the below code,
<asp:TextBox ID="txtName"
runat="server"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator1" runat="server"
ControlToValidate="txtName" ErrorMessage="Name is
mandatory."></asp:RequiredFieldValidator>
Using the RequiredFieldValidator to
validate a DropDownList is bit different when compared to TextBox control. The
below code does that.
<asp:DropDownList ID="ddlCountry"
runat="server">
<asp:ListItem>Select</asp:ListItem>
<asp:ListItem>India</asp:ListItem>
<asp:ListItem>US</asp:ListItem>
<asp:ListItem>UK</asp:ListItem>
</asp:DropDownList>
<asp:RequiredFieldValidator
ID="RequiredFieldValidator1"
InitialValue="Select"
runat="server"
ControlToValidate="ddlCountry"
ErrorMessage="RequiredFieldValidator"></asp:RequiredFieldValidator>
The only difference is we need to set
the InitialValue property of the validator control to the default selected value
of the DropDownList.
RegularExpressionValidator
control
Sometimes, we may require validating
inputs based on some patterns i.e. the input should be in particular format. For
example, date format validations, email format, etc. These types of validations
can be done using regular expression and javascript in ASP days. In ASP.Net, we
can use the RegularExpressionValidator control which accepts a regular
expression to validate the input.
Refer the below code.
<asp:TextBox ID="txtEmail"
runat="server"></asp:TextBox>
<asp:RegularExpressionValidator ID="RegularExpressionValidator1"
runat="server"
ControlToValidate="txtEmail" ErrorMessage="Please enter a valid email"
ValidationExpression="\w+([-+.']\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*"></asp:RegularExpressionValidator>
When we use Visual Studio, there are
some predefined validation expressions available for the most often used input
patterns like email, web address, PIN code, etc. Refer the below
figure.
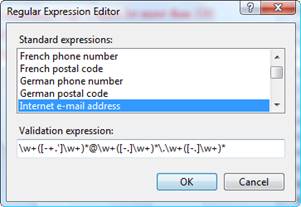
Click the button you find
ValidationExpression property in property window of Visual studio to enable
above dialog.
RangeValidator control
This validator control will help us to
validate an input control to accept the value which should fall in a particular
range. For example, if we want to restrict age textbox to fall within a range 1
to 100.
Refer the below code,
<asp:TextBox ID="txtAge"
runat="server"></asp:TextBox>
<asp:RangeValidator
ID="RangeValidator1" runat="server"
ControlToValidate="txtAge"
ErrorMessage="Age Cannot be more than 100"
MaximumValue="100" MinimumValue="1"
Type="Integer"></asp:RangeValidator>
To make the validator work we need to
set a property called Type(for specifying the type of the input).
CompareValidator control
This validator control will help us to
compare the input in 2 input controls for a match like equal, less than, greater
than, etc. For example, password and confirm password field match.
To validate a email and confirm email
field to have same email typed by the user,
<asp:TextBox ID="txtEmail"
runat="server"></asp:TextBox>
<asp:TextBox ID="txtConfirmEmail"
runat="server"></asp:TextBox>
<asp:CompareValidator
ID="CompareValidator2" runat="server"
ControlToCompare="txtEmail"
ControlToValidate="txtConfirmEmail"
ErrorMessage="Emails not
matching"></asp:CompareValidator>
|