Moving forward, we will see how to create zip files on
the fly using the free component dotnetzip in ASP.Net projects.
According to the official page,
DotNetZip is an easy-to-use, FAST, FREE class library
and toolset for manipulating zip files or folders. Zip and Unzip is easy: with
DotNetZip, .NET applications written in VB, C# - any .NET language - can easily
create, read, extract, or update zip files. For Mono or MS .NET.
Steps
1. In your Visual Studio 2008, click File > New > WebSite. In the "New Web Site" dialog, Select "Asp.Net WebSite". In the Language DropDownlist at the bottom, choose C#.
You can specify the name of the project in Location textbox.
2. Download the latest dotnetzip component. The latest
version as of this writing is v1.9.
3. Unzip the
DotNetZipLib-DevKit-v1.9.zip file. You can see unzipped folder contents as
below.
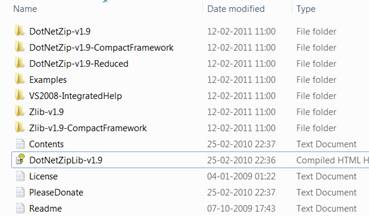
Navigate to DotNetZip-v1.9 folder where you will the
.dll called Ionic.Zip.dll in Debug and Release folder. This is the assembly
which is required to generate the zip files. Right click your project in the
solution explorer and click “Add reference..”. Go to Browse tab and select the
Ionic.Zip.dll in Release folder and click OK.
4. For easy understanding purpose,
we will copy some image files in the solution under the folder “Albums\Jokers”.
We will use this content in our sample application to zip the content using
dotnetzip component and send it to client. The solution will look like,
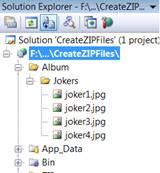
Please remember, compressing any media files will not
give you better results. I have just taken it as an example; you can use any
text files or other files to see the difference. This article will just help you
to use dotnetzip component for creating zip files on the fly in ASP.Net and will
not deal with the actual compression techniques in detail.
5. We will create a simple UI by
displaying all the files found in the folder Albums\Jokers by displaying a
checkbox near it. User can select the file and click the “Download as zip file”
button to download it as a zip file. See the image below,
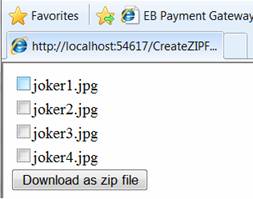
Below is the code used to build the above UI.
ASPX
<form id="form1" runat="server">
<div>
<asp:CheckBoxList ID="chkAlbum"
runat="server">
</asp:CheckBoxList>
<asp:Button ID="btnDownload" runat="server"
onclick="btnDownload_Click"
Text="Download as zip file" />
</div>
CodeBehind
protected void Page_Load(object sender, EventArgs e)
{
string[] files =
Directory.GetFiles(Server.MapPath(".") + @"\Album\Jokers”);
foreach (string file in files)
{
chkAlbum.Items.Add(new
ListItem(Path.GetFileName(file), file));
}
}
In the above code, i have used a CheckBoxList control to
populate the files under Albums\Jokers folder. The text will show the file name
and the value will hold the full path of the file.
In the coming sections, we will see how to zip the
contents based on the user selection.
Creating Zip files at Runtime
To zip the selected files, we need to iterate over the
Item collection of CheckBoxList control and check its “Selected” property. This
will be true if the item is selected. We can include it in the zip file we
create if the Selected is true. The dotnetzip component has a class called
ZipFile which can be used to create the zip files. See the below code,
protected void btnDownload_Click(object sender, EventArgs
e)
{
Response.Clear();
Response.ContentType = "application/zip";
Response.AddHeader("content-disposition",
"filename=" + "Album.zip");
using (ZipFile zip = new ZipFile())
{
foreach (ListItem item in chkAlbum.Items)
{
if (item.Selected)
{
zip.AddFile(item.Value,"Album");
}
}
zip.Save(Response.OutputStream);
}
}
In the above code, we have used ZipFile.AddFile() method
to add it to the zip file we are creating. The first parameter is the path of
the file we are adding to zip and second is an optional parameter which will
dictate the folder name under which the file will be archived in the zip file.
If we didn’t supply the second parameter, the file will be created in the same
hierarchy of the folders as it is found in the original file system in the zip
file. That’s it! Execute the page and see it in action.
Zip entire folder
The code in the previous section helped to zip the files
by adding them one by one. The dotnetzip component allows us to add a folder or
directory instead of adding files individually using the ZipFile.AddDirectory()
method. To this, drag another button and name it as btnDownLoadDir. See the
below code,
protected void btnDownLoadDir_Click(object sender,
EventArgs e)
{
Response.Clear();
Response.ContentType = "application/zip";
Response.AddHeader("content-disposition",
"filename=" + "Album.zip");
using (ZipFile zip = new ZipFile())
{
zip.AddDirectory(Server.MapPath(".") +
@"\Album\Jokers");
zip.Save(Response.OutputStream);
}
}
|