2. Bind the ListView from the CodeBehind using LINQ query and LINQ to
SQL classes.
protected void Page_Load(object sender,
EventArgs e)
{
if (!IsPostBack)
{
BindEmp();
BindDeptDDL();
}
}
public void BindEmp()
{
EmployeeInfoDataContext dbEmp =
new EmployeeInfoDataContext();
var LINQQuery = from emp in
dbEmp.Employees
select
emp;
lvEmployee.DataSource =
LINQQuery;
lvEmployee.DataBind();
}
public void BindDeptDDL()
{
DropDownList ddl = lvEmployee.InsertItem.FindControl("DropDownList1") as DropDownList;
ddl.DataSource = BindDept();
ddl.DataBind();
}
public IEnumerable
BindDept()
{
EmployeeInfoDataContext dbEmp =
new EmployeeInfoDataContext();
var LINQQuery = from dept in
dbEmp.Departments
select
dept;
return LINQQuery as
IEnumerable;
}
Execute the page; you can see ListView
with employee records.
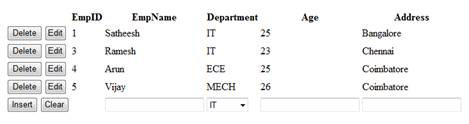
3. For ListView edit, delete and insert command to work we need to write
custom LINQ query and execute it using LINQ to SQL classes. The custom LINQ
queries are bolded in the below code.
protected void
lvEmployee_ItemEditing(object sender, ListViewEditEventArgs e)
{
lvEmployee.EditIndex =
e.NewEditIndex;
BindEmp();
BindDeptDDL();
}
protected void
lvEmployee_ItemCanceling(object sender, ListViewCancelEventArgs e)
{
lvEmployee.EditIndex =
-1;
BindEmp();
BindDeptDDL();
}
protected void
lvEmployee_ItemUpdating(object sender, ListViewUpdateEventArgs e)
{
EmployeeInfoDataContext dbEmp =
new EmployeeInfoDataContext();
Label lblTemp =
lvEmployee.Items[e.ItemIndex].FindControl("EmpIDLabel1") as Label;
string EmpID = "0";
if(lblTemp !=null)
EmpID =
lblTemp.Text;
Employee empTemp = (from emp in
dbEmp.Employees
where
emp.EmpID == int.Parse(EmpID)
select
emp).Single();
TextBox txtTemp =
lvEmployee.Items[e.ItemIndex].FindControl("EmpNameTextBox") as
TextBox;
if (txtTemp != null)
empTemp.EmpName =
txtTemp.Text;
DropDownList ddlTemp =
lvEmployee.Items[e.ItemIndex].FindControl("DropDownList2") as
DropDownList;
if (txtTemp != null)
empTemp.DeptID =
int.Parse(ddlTemp.SelectedValue);
txtTemp =
lvEmployee.Items[e.ItemIndex].FindControl("AgeTextBox") as TextBox;
if (txtTemp != null)
empTemp.Age =
int.Parse(txtTemp.Text);
txtTemp =
lvEmployee.Items[e.ItemIndex].FindControl("AddressTextBox") as
TextBox;
if (txtTemp != null)
empTemp.Address =
txtTemp.Text;
dbEmp.SubmitChanges();
lvEmployee.EditIndex =
-1;
BindEmp();
BindDeptDDL();
}
protected void
lvEmployee_ItemDeleting(object sender, ListViewDeleteEventArgs e)
{
EmployeeInfoDataContext dbEmp =
new EmployeeInfoDataContext();
Label lblTemp =
lvEmployee.Items[e.ItemIndex].FindControl("EmpIDLabel") as Label;
string EmpID = "0";
if(lblTemp !=null)
EmpID =
lblTemp.Text;
Employee empTemp = (from emp in
dbEmp.Employees
where
emp.EmpID == int.Parse(EmpID)
select
emp).Single();
dbEmp.Employees.DeleteOnSubmit(empTemp);
dbEmp.SubmitChanges();
BindEmp();
BindDeptDDL();
}
protected void
lvEmployee_ItemInserting(object sender, ListViewInsertEventArgs e)
{
EmployeeInfoDataContext dbEmp =
new EmployeeInfoDataContext();
Employee emp = new
Employee();
TextBox txtTemp =
e.Item.FindControl("EmpNameTextBox") as TextBox;
emp.EmpName =
txtTemp.Text;
DropDownList ddlTemp =
e.Item.FindControl("DropDownList1") as DropDownList;
emp.DeptID =
int.Parse(ddlTemp.SelectedValue);
txtTemp =
e.Item.FindControl("AgeTextBox") as TextBox;
emp.Age =
int.Parse(txtTemp.Text);
txtTemp =
e.Item.FindControl("AddressTextBox") as TextBox;
emp.Address =
txtTemp.Text;
dbEmp.Employees.InsertOnSubmit(emp);
dbEmp.SubmitChanges();
BindEmp();
BindDeptDDL();
}
Execute the page and see ListView
edit/update/delete/insert in action using LINQ.
Note
The example discussed in this article uses lazy loading to load the related data(DepartmentName). Read the article
Using DataLoadOptions to Fetch(Immediate Load) the Related Objects in LINQ
to reduce the database rountrips or immediately load the related data.
|