Step 6:
Add the following code in the Load event handler of MyControl after declaring an
array for storing a list of names of the cities.
string[] cities;
private void MyControl_Load(object sender,
EventArgs e)
{
cities = new string[] { "NewYork",
"Mumbai", "Belgrade", "Los Angeles", "Chennai", "New Delhi", "Madrid",
"Chicago","Las Vegas", "Bangalore", "Sydney", "Melbourne", "Beijing",
"Calcutta", "Milan", "Austin", "Brisbane" };
}
Step 7:
In the TextChanged event handler for the label1 control, add the following code
that filters the names of the cities and populate the list box. It uses a LINQ
query on the cities array and filters the names that start with the typed
characters in the Smart Tag Panel. It is to note that the label1 is updated when
the LabelText property of Mycontrol is changed.
private void
label1_TextChanged(object sender, EventArgs e)
{
listBox1.Items.Clear();
IEnumerable<string>
filtered =
from a in
cities
where
a.StartsWith(label1.Text)
orderby
a
select a;
if (filtered.Count() >
0)
listBox1.Items.AddRange(filtered.ToArray());
}
Step 8:
Now, ControlDesigner class must be defined for MyControl as specified in the
attribute in Step 5.This class myControlDesigner is attached with the
PermissionSet attribute as it requires a full permission to access Smart Tag
features.
[System.Security.Permissions.PermissionSet(System.Security.Permissions.SecurityAction.Demand,
Name = "FullTrust")]
public class myControlDesigner :
System.Windows.Forms.Design.ControlDesigner
{
private
DesignerActionListCollection actionLists;
// Use pull model to populate
smart tag menu.
public override
DesignerActionListCollection ActionLists
{
get
{
if (null ==
actionLists)
{
actionLists = new
DesignerActionListCollection();
actionLists.Add(new
myControlActionList(this.Component));
}
return
actionLists;
}
}
}
Step 9:
The following code creates a list of Smart Tag menu actions/commands for the
usercontrol as specified in its constructor. The DesignerActionList class is
inherited and the methods are implemented to created Smart Tag items.
public class myControlActionList :
System.ComponentModel.Design.DesignerActionList
{
private MyControl
colUserControl;
private DesignerActionUIService
designerActionUISvc = null;
//The constructor associates the
control with the smart tag list.
public
myControlActionList(IComponent component)
: base(component)
{
this.colUserControl =
component as MyControl;
// Cache a reference to
DesignerActionUIService, so the DesigneractionList can be refreshed.
this.designerActionUISvc =
GetService(typeof(DesignerActionUIService)) as
DesignerActionUIService;
}
.....
.....
// Implementation of this abstract
method creates smart tag items, associates their targets, and collects into
list.
public override
DesignerActionItemCollection GetSortedActionItems()
{
DesignerActionItemCollection
items = new DesignerActionItemCollection();
//Define static section header
entries.
items.Add(new
DesignerActionHeaderItem("Appearance"));
items.Add(new
DesignerActionPropertyItem("BackColor",
"Back
Color", "Appearance",
"Selects
the background color."));
items.Add(new
DesignerActionPropertyItem("ForeColor",
"Fore
Color", "Appearance",
"Selects
the foreground color."));
items.Add(new
DesignerActionPropertyItem("LabelText",
"Label
Text", "Appearance",
"Type few
characters to filter Cities."));
return items;
}
Step 10:
Build the project.
Step 11:
Open Form1 designer and drag the usercontrol “MyControl” in the
toolbox to the designer surface and see Smart Tag working smoothly.
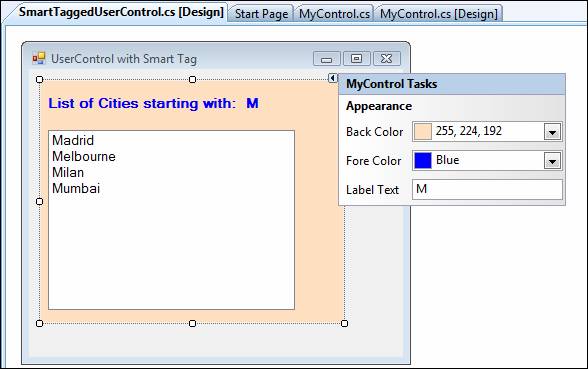
Note: Complete code for creating a
usercontrol with smart tag explained in this sample application is available for
download along with this article. You may add the file MyControl.cs file
to any Windows Forms application and add reference to System.Design
assembly before building the project. Place the usercontrol in any form and see
Smart Tag features working for you.
|