Since, developing a component ourselves
is complicated and time consuming task we can try using any free 3rd
party component available in the market. In this article, we will try using one
of most widely used PDF generation component called iTextSharp
or iText
PDF component.
According to iText PDF
official site,
iText is a library that allows you
to generate PDF files on the fly.
iText is an ideal library for
developers looking to enhance web- and other applications with dynamic PDF
document generation and/or manipulation.
In this article, we will see
about,
1. Simple PDF generation from an in-memory string or HTML
string
2. Export Page Content to PDF
3. Export GridView control to PDF
Steps
1. Go to Start> All Programs> Open Visual Studio 2008. Create a new test Asp.Net project. Select your familiar language,
i have used C# to demonstrate in this article.
2. Download the iText PDF component from the official site (or
from here) and add it to your project through add
reference dialog box.
Simple PDF generation from an in-memory
String or HTML String
Sometimes, we may need to generate PDF
file on the fly from a string value obtained from database, etc.
The below code will help you to do
that,
protected void btnCreate_Click(object
sender, EventArgs e)
{
string str ="I Love ASP.Net!";
Document document = new
Document();
PdfWriter.GetInstance(document,
new FileStream(@"C:\Temp\test.pdf", FileMode.Create));
document.Open();
Paragraph P = new Paragraph(str,
FontFactory.GetFont("Arial", 10));
document.Add(P);
document.Close();
}
The above code will generate a PDF file
called test.pdf with the string "I Love ASP.Net!".
Note
Your service account should have proper
access to the report generation path in order to save the report.
You can also add text through an object
called Chunk packed inside iTextSharp.text namespace. Refer below,
protected void btnCreate_Click(object
sender, EventArgs e)
{
string str ="I Love ASP.Net!";
Document document = new
Document();
PdfWriter.GetInstance(document,
new FileStream(@"C:\Temp\test.pdf", FileMode.Create));
document.Open();
Paragraph P = new Paragraph(str,
FontFactory.GetFont("Arial", 10));
Chunk ch = new Chunk("ASP.Net is a
great technology!",FontFactory.GetFont("Arial",6));
P.Add(ch);
document.Add(P);
document.Close();
}
When executed, the PDF report will look
like,
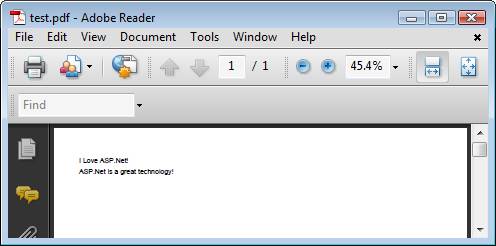
To generate PDF file from HTML content
we have to use a class called HTMLWorker available in iTextSharp.text.html
namespace.
Refer the below code,
protected void btnHTMLCreate_Click(object
sender, EventArgs e)
{
Document document = new
Document();
PdfWriter.GetInstance(document,
new FileStream(@"C:\Temp\test.pdf", FileMode.Create));
document.Open();
string strHTML = "<B>I Love
ASP.Net!</B>";
HTMLWorker htmlWorker = new
HTMLWorker(document);
htmlWorker.Parse(new
StringReader(strHTML));
document.Close();
}
The above code will generate PDF report
by applying the HTML styles.
Refer below,
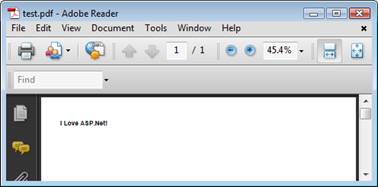
You have inlcude the below namespace in
your page for the above codes to work.
using iTextSharp.text;
using iTextSharp.text.pdf;
using iTextSharp.text.html;
using
iTextSharp.text.html.simpleparser;
Export Page Content to PDF
At times, we may need to export the
page content to a PDF report. For example, providing an option in an article
site to download the article in PDF format for future reference. Assuming, the
content you want to export to PDF is inside DIV tag(dvTExt) or Panel, the below
code will export the content to a PDF file.
ASPX
<div id="dvText"
runat="server">
//Content goes here
</div>
CodeBehind
protected void btnExport_Click(object
sender, EventArgs e)
{
string attachment = "attachment;
filename=Article.pdf";
Response.ClearContent();
Response.AddHeader("content-disposition", attachment);
Response.ContentType =
"application/pdf";
StringWriter stw = new
StringWriter();
HtmlTextWriter htextw = new
HtmlTextWriter(stw);
dvText.RenderControl(htextw);
Document document = new
Document();
PdfWriter.GetInstance(document,
Response.OutputStream);
document.Open();
StringReader str = new
StringReader(stw.ToString());
HTMLWorker htmlworker = new
HTMLWorker(document);
htmlworker.Parse(str);
document.Close();
Response.Write(document);
Response.End();
}
|