To do the transformation, we need to
define a set of transformation rules using XSL (Extensible Stylesheet Language)
language. i.e. to convert or transform a xml file to HTML we need to define
transformation rules which dictates the XSLT engine to convert a XML node or
element to a output format(in HTML/XHTML/ or XML). Hence, to transform a XML
file we need to define xsl file which has some conversion rules. We will
understand this clearly in later part of this article.
To understand this easily, we will use
sample xml file that contains employee information’s. Refer below,
<?xml version="1.0"
encoding="utf-8"?>
<Employees>
<Employee>
<ID>100</ID>
<FirstName>Bala</FirstName>
<LastName>Murugan</LastName>
<Dept>Production
Support</Dept>
</Employee>
<Employee>
<ID>101</ID>
<FirstName>Peter</FirstName>
<LastName>Laurence</LastName>
<Dept>Development</Dept>
</Employee>
<Employee>
<ID>102</ID>
<FirstName>Rick</FirstName>
<LastName>Anderson</LastName>
<Dept>Sales</Dept>
</Employee>
<Employee>
<ID>103</ID>
<FirstName>Ramesh</FirstName>
<LastName>Kumar</LastName>
<Dept>HR</Dept>
</Employee>
<Employee>
<ID>104</ID>
<FirstName>Katie</FirstName>
<LastName>Yu</LastName>
<Dept>Recruitment</Dept>
</Employee>
<Employee>
<ID>105</ID>
<FirstName>Suresh</FirstName>
<LastName>Babu</LastName>
<Dept>Inventory</Dept>
</Employee>
</Employees>
Assume, you want to display the above
XML data in a browser (something similar to below figure).
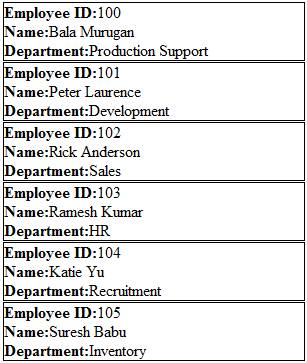
To do this, we will first develop a XSL
file that has some rules to transform the XML data. XSL language will use XPATH
query to navigate through node elements and attributes in XML documents. Also,
it includes for-each loops, if statement, etc similar to programming languages
to assist the transformations.
The below xsl file will select each
employee node and emits the HTML specified for the match. Refer
below,
EmployeeXSLT.xslt
<?xml version="1.0"
encoding="iso-8859-1"?>
<xsl:stylesheet version="1.0"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/">
<xsl:for-each
select="//Employee">
<div style="border:1px black
solid;width:300px;margin:1px">
<div>
<b>Employee ID:</b>
<xsl:value-of select="ID"/>
</div>
<div>
<b>Name:</b>
<xsl:value-of select="FirstName"/><xsl:text>
</xsl:text><xsl:value-of select="LastName"/>
</div>
<div>
<b>Department:</b>
<xsl:value-of select="Dept"/>
</div>
</div>
</xsl:for-each>
</xsl:template>
</xsl:stylesheet>
In the above code, <xsl:template
match="/"> will return the root element (Employees) in XML file. The
//employee will select all the occurrence of employee node under the root
element <Employees> and converts the xml to HTML.
To know more XSL and the syntax refer
here.
XSLT Transformation using
ASP.Net
.Netframework includes a class called
XslCompiledTransform in System.Xml.Xsl namespace which can be used to perform
the transformation. In specific, the XslCompiledTransform class is packed with a
method called XslCompiledTransform.Transform() which can be used to the
transformation.
The below code can be used to trasform
a xml data from an XML file (Employess.xml) using the XSLT
file(EmployeeXSLT.xslt).
Refer the below code,
CodeBehind
protected void Page_Load(object sender,
EventArgs e)
{
string strXSLTFile =
Server.MapPath("EmployeeXSLT.xslt");
string strXMLFile =
Server.MapPath("App_Data/Employess.xml");
XmlReader reader =
XmlReader.Create(strXMLFile);
XslCompiledTransform
objXSLTransform = new XslCompiledTransform();
objXSLTransform.Load(strXSLTFile);
StringBuilder htmlOutput = new
StringBuilder();
TextWriter htmlWriter = new
StringWriter(htmlOutput);
objXSLTransform.Transform(reader,
null, htmlWriter);
ltRss.Text =
htmlOutput.ToString();
reader.Close();
}
ASPX
<div>
<asp:Literal ID="ltRss"
runat="server"></asp:Literal>
</div>
In the above code, the transformed HTML
output is displayed in the browser using a Literal control(ltRss).
At times, we will require to transform
xml data read from database or from other datasources. The below code does
that,
|