Enabling Select All
If we see the rendered output of the GridView, it will
be rendered as a HTML Table with ID as GridView ID.
Refer the below output.
<table cellspacing="0" cellpadding="4" rules="all"
border="1" id="gvUsers"
style="background-color:White;border-color:#010101;border-width:1px;border-style:Groove;border-collapse:collapse;">
<tr
style="color:#FFFFCC;background-color:#F06300;font-weight:bold;">
<th scope="col">
<input
id="gvUsers_ctl01_chkAll" type="checkbox" name="gvUsers$ctl01$chkAll"
onclick="javascript:SelectAllCheckboxesSpecific(this);" />
</th><th
scope="col">Email</th><th scope="col">First Name</th><th
scope="col">Last Name</th>
</tr><tr
style="color:#330099;background-color:White;">
<td>
<input
id="gvUsers_ctl02_chkDelete" type="checkbox" name="gvUsers$ctl02$chkDelete"
onclick="javascript:HighlightRow(this);" />
</td><td>test1@gmail.com</td><td>Babu</td><td>B</td>
//Goes on
So, we will first find the table from the rendered HTML
and get all the checkbox inside table to check/uncheck depending on the state of
the CheckBox control in header. Refer the below JavaScript function which
selects all the checkbox inside the GridView when the Header CheckBox control is
checked.
function SelectAllCheckboxesSpecific(spanChk)
{
var IsChecked = spanChk.checked;
var Chk = spanChk;
Parent =
document.getElementById('gvUsers');
var items =
Parent.getElementsByTagName('input');
for(i=0;i<items.length;i++)
{
if(items[i].id != Chk &&
items[i].type=="checkbox")
{
if(items[i].checked!= IsChecked)
{
items[i].click();
}
}
}
}
Executing the page and see it in action.
Since, we are getting all the elements with tag name as
“input”, the above code will check/uncheck the other CheckBox controls if
present in any other column of the GridView control. To understand it better,
include a checkbox at the last and execute the page. It will Check/Uncheck for
the actions you do in the Header CheckBox. If you don’t have another checkbox
control in the GridView, the above JavaScript code will work fine.
Refer the below figure.
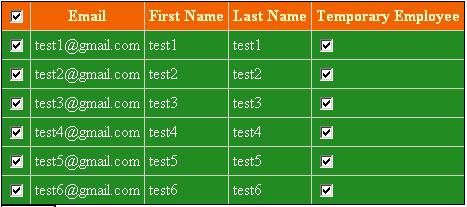
To handle the above scenario with multiple CheckBox, we
will change the code bit to check/uncheck the CheckBox control found based on
the column location instead of checking all the CheckBox in the GridView. If we
see the rendered HTML table, we have the checkbox at every first TD of the
table. We will find the checkbox in every first TD and perform the required
action. The below code does that.
function SelectAllCheckboxesMoreSpecific(spanChk)
{
var IsChecked = spanChk.checked;
var Chk = spanChk;
Parent =
document.getElementById('gvUsers');
for(i=0;i< Parent.rows.length;i++)
{
var tr = Parent.rows[i];
//var td =
tr.childNodes[0];
var td = tr.firstChild;
var item = td.firstChild;
if(item.id != Chk &&
item.type=="checkbox")
{
if(item.checked!= IsChecked)
{
item.click();
}
}
}
}
Configure the onclick event of header checkbox to point
to the above JavaScript function.
The above code assumes the checkbox will be the first
column of every row in the GridView. If the GridView have the selection CheckBox
as the last column, then change the line which is bolded above to var item =
td.lastChild;
This code will work perfectly even if there are CheckBox
controls present in any other column of the GridView control.
We can include a button that deletes the selected
row,
protected void btnDelete_Click(object sender, EventArgs
e)
{
for (int i = 0; i < gvUsers.Rows.Count;i++ )
{
CheckBox chbTemp =
gvUsers.Rows[i].FindControl("chkDelete") as CheckBox;
if (chbTemp.Checked)
{
Response.Write(gvUsers.Rows[i].Cells[1].Text + "<BR>");
//Code for Deletion
}
}
}
I took the example to delete the selected rows; it can
be any operation that is specific to your requirement that can be done on the
selected rows.
|