Pre-Requisites
Visit the following link to download the latest Ajax
Control Toolkit.
http://www.asp.net/AJAX/ajaxcontroltoolkit/
You can download this package with or without source
code. Download it and unzip the package.
For ASP.Net AJAX 1.0 and Visual Studio 2005, you can
download the toolkit directly from here.
Read my previous article, Ajax
Control Toolkit – Introduction , to configure Ajax Control Toolkit with
Visual Studio 2005.
Sharepoint List Style GridView
We will normally have separate columns for edit, delete
and select in GridView. In this article, we will provide edit, delete and select
links in DropDownExtender control which will be displayed when the user clicks
the first column of the GridView, similar to sharepoint list. By doing this, we
can reduce the space occupied by the GridView horizontally and also it will give
a better user experience. Refer the below figure.
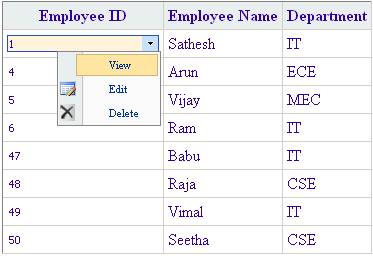
Constructing the GridView
1. Drag an UpdatePanel
Control.
2. Drag a GridView Control.
3. Make Employee Name and
Department as a BoundColumn. Employee ID column should be a TemplateColumn to
include extender controls.
4. In the Employee ID template
column, include a Label control to display the Employee ID. Again, include a
panel control with 3 LinkButtons for View, Edit and Delete operation.
To make this panel to appear as a dropdown when the user
clicks on the Employee ID, include a DropDownExtender control from Ajax Control
Toolkit toolbar. Configure its TargetControlID property to EmployeeID label
control and DropDownControlID property to the panel that contains the
linkbuttons.
We will also include a ConfirmButtonExtender to give a
confirm box when the user click Delete option.
The final GridView will look like,
<asp:GridView ID="GridView1" runat="server"
BackColor="White"
BorderColor="#CC9966" BorderStyle="None" BorderWidth="1px"
CellPadding="4"
AutoGenerateColumns="False"
OnRowCommand="GridView1_RowCommand">
<FooterStyle BackColor="#FFFFCC" ForeColor="#330099"
/>
<RowStyle BackColor="White" ForeColor="#330099"
/>
<SelectedRowStyle BackColor="#FFCC66" Font-Bold="True"
ForeColor="#663399" />
<PagerStyle BackColor="#FFFFCC" ForeColor="#330099"
HorizontalAlign="Center" />
<HeaderStyle BackColor="#e9eeee" Font-Bold="True"
ForeColor="#330099" />
<Columns>
<asp:TemplateField HeaderText="Employee
ID">
<ItemTemplate>
<asp:Label ID="lblEmpID" runat="server"
Text='<%# Eval("EmpID") %>'
Style="display:
block;padding:2px;width:100px;padding-right: 50px; font-family: Tahoma;
font-size: 11px;" />
<asp:Panel ID="DropPanel" runat="server"
CssClass="ContextMenuPanel" Style="width:150px;display :none; visibility:
hidden;">
<table cellpadding="0"
cellspacing="0">
<tr><td></td><td>
<asp:LinkButton ID="lbView"
runat="server" CssClass="ContextMenuItem"
CommandName="View">View</asp:LinkButton></td></tr>
<tr><td><img src="Images/edit.png"
/></td>
<td><asp:LinkButton runat="server"
CommandName="EditRow" ID="lbEdit" Text="Edit" CssClass="ContextMenuItem"
/></td>
</tr>
<tr><td><img
src="Images/delete.png" /></td>
<td><asp:LinkButton runat="server"
ID="lbDelete" CommandName="DeleteRow" Text="Delete" CssClass="ContextMenuItem"
/></td>
</table>
</asp:Panel>
<ajaxToolkit:DropDownExtender runat="server"
ID="extOperation"
TargetControlID="lblEmpID"
DropDownControlID="DropPanel" />
<ajaxToolkit:ConfirmButtonExtender
ID="ConfirmButtonExtender1" ConfirmText="Are you sure to delete?"
TargetControlID="lbDelete" runat="server">
</ajaxToolkit:ConfirmButtonExtender>
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="EmpName"
HeaderText="Employee Name" SortExpression="EmpName" />
<asp:BoundField DataField="Department"
HeaderText="Department" SortExpression="Department" />
</Columns>
</asp:GridView>
Codebehind
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
string query = "SELECT * FROM [Employee]";
GridView1.DataSource = GetDt(query);
GridView1.DataBind();
}
}
public DataTable GetDt(string query)
{
SqlConnection con = new
SqlConnection(ConfigurationManager.ConnectionStrings["ConnectionString"].ConnectionString);
SqlDataAdapter ada = new SqlDataAdapter(query,
con);
DataTable dt = new DataTable();
ada.Fill(dt);
return dt;
}
|