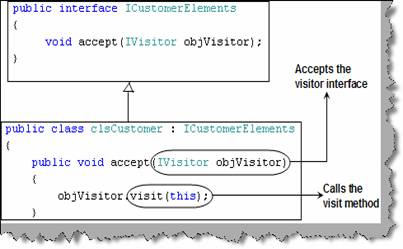
Figure: -
Visitor passed to data structure class
Now every customer has multiple address objects and
every address has multiple phone objects. So we have ‘objAddresses’ arraylist
object aggregated in the ‘clsCustomer’ class and ‘objPhones’ arraylist
aggregated in the ‘clsAddress’ class. Every object has the accept method which
takes the visitor class and passes himself in the visit function of the visitor
class. As the visit function of the visitor class is overloaded it will call the
appropriate visitor method as per polymorphism.
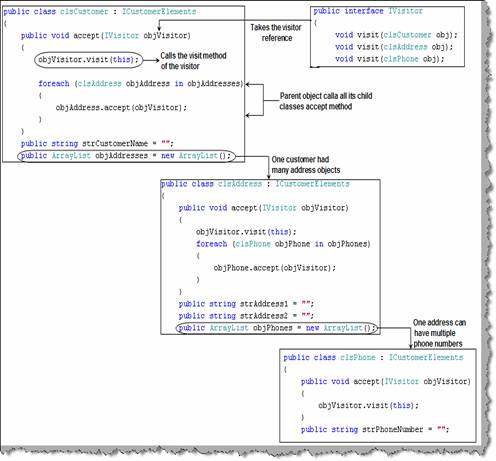
Figure: -
Customer, Address and phones
Now that we have the logic in the visitor classes and
data structure in the customer classes its
time to use the same in the client. Below figure
‘Visitor client code’ shows a sample code snippet for using the visitor pattern.
So we create the visitor object and pass it to the customer data class. If we
want to display the customer object structure in a string format we create the
‘clsVisitorString’ and if we want to generate in XML format we create the
‘clsXML’ object and pass the same to the customer object data structure. You can
easily see how the logic is now separated from the data structure.
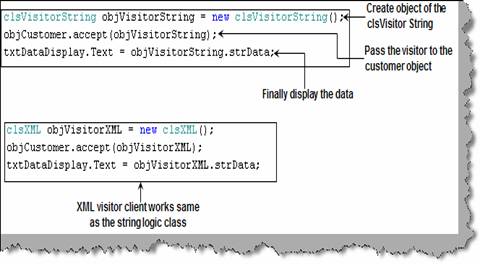
Figure: -
Visitor client code
Note: - You can find a sample of
the same in C# in the visitor folder of the CD. If you belong to some other
programming domain you can map the same as the code is very
generic.
(A) What the difference between visitor and strategy
pattern?
Visitor and strategy look very much similar as they deal
with encapsulating complex logic from data. We can say visitor is more general
form of strategy.
In strategy we have one context or a single logical data
on which multiple algorithms operate. In the previous questions we have
explained the fundamentals of strategy and visitor. So let’s understand the same
by using examples which we have understood previously. In strategy we have a
single context and multiple algorithms work on it. Figure ‘Strategy’ shows how
we have a one data context and multiple algorithm work on it.
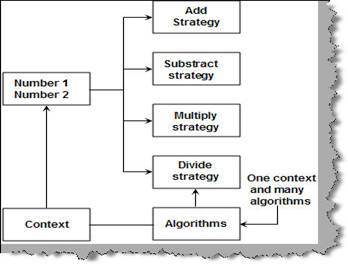
Figure: -
Strategy
In visitor we have multiple contexts and for every
context we have an algorithm. If you remember the visitor example we had written
parsing logic for every data context i.e. customer, address and phones
object.
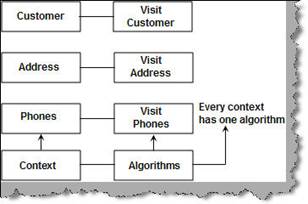
Figure: -
Visitor
So in short strategy is a special kind of visitor. In
strategy we have one data context and multiple algorithms while in visitor for
every data context we have one algorithm associated. The basic criteria of
choosing whether to implement strategy or visitor depends on the relationship
between context and algorithm. If there is one context and multiple algorithms
then we go for strategy. If we have multiple contexts and multiple algorithms
then we implement visitor algorithm.
(A) Can you explain adapter
pattern?
Many times two classes are incompatible because of
incompatible interfaces. Adapter helps us to wrap a class around the existing
class and make the classes compatible with each other. Consider the below figure
‘Incompatible interfaces’ both of them are collections to hold string values.
Both of them have a method which helps us to add string in to the collection.
One of the methods is named as ‘Add’ and the other as ‘Push’. One of them uses
the collection object and the other the stack. We want to make the stack object
compatible with the collection object.
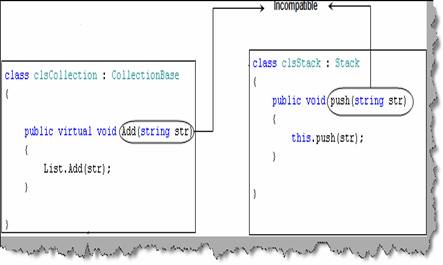
Figure: -
Incompatible interfaces
There are two way of implementing adapter pattern one is
by using aggregation (this is termed as the object adapter pattern) and the
other inheritance (this is termed as the class adapter pattern). First let’s try
to cover object adapter pattern.
Figure ‘Object Adapter pattern’ shows a broader view of
how we can achieve the same. We have a introduced a new wrapper class
‘clsCollectionAdapter’ which wraps on the top of the ‘clsStack’ class and
aggregates the ‘push’ method inside a new ‘Add’ method, thus making both the
classes compatible.
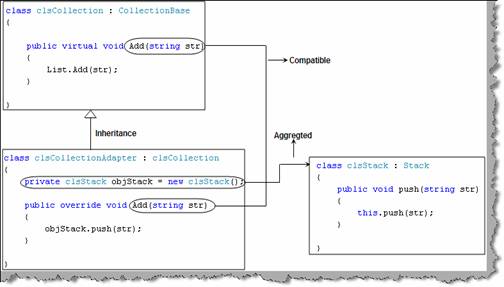
Figure: -
Object Adapter pattern
The other way to implement the adapter pattern is by
using inheritance also termed as class adapter pattern. Figure ‘Class adapter
pattern’ shows how we have inherited the ‘clsStack’ class in the
‘clsCollectionAdapter’ and made it compatible with the ‘clsCollection’
class.
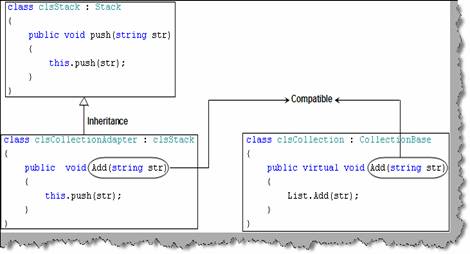
Figure :- Class
adapter pattern
Note :- You can the above C#
example in the adapter folder of the CD.
(I) What is fly weight
pattern?
Fly weight pattern is useful where we need to create
many objects and all these objects share some kind of common data. Consider
figure ‘Objects and common data’. We need to print visiting card for all
employees in the organization. So we have two parts of data one is the variable
data i.e. the employee name and the other is static data i.e. address. We can
minimize memory by just keeping one copy of the static data and referencing the
same data in all objects of variable data. So we create different copies of
variable data, but reference the same copy of static data. With this we can
optimally use the memory.
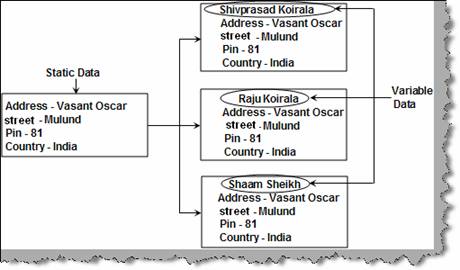
Figure: - Objects and common data
Below is a sample C# code demonstration of how flyweight
can be implemented practically. We have two classes, ‘clsVariableAddress’ which
has the variable data and second ‘clsAddress’ which has the static data. To
ensure that we have only one instance of ‘clsAddress’ we have made a wrapper
class ‘clsStatic’ and created a static instance of the ‘clsAddress’ class. This
object is aggregated in the ‘clsVariableAddress’ class.
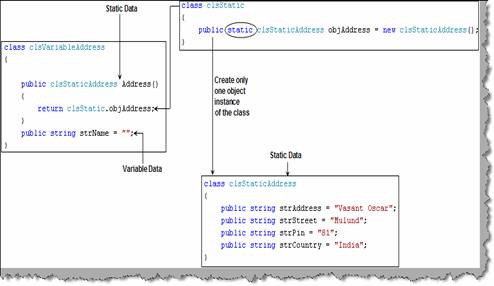
Figure: -
Class view of flyweight
Figure ‘Fly weight client code’ shows we have created
two objects of ‘clsVariableAddress’ class, but internally the static data i.e.
the address is referred to only one instance.
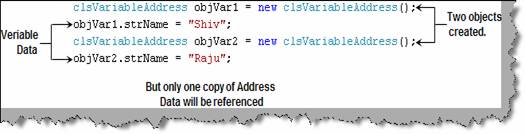
Figure: - Fly
weight client code
|