To give you a practical understanding i have put all
these design patterns in a video format and uploaded here. You can visit questpond.com and download the complete
architecture interview questions PDF which covers SOA , UML , Design patterns ,
Togaf , OOPs etc.
What are design patterns?
Design patterns are documented tried and tested
solutions for recurring problems in a given context. So basically you have a
problem context and the proposed solution for the same. Design patterns existed
in some or other form right from the inception stage of software development.
Let’s say if you want to implement a sorting algorithm the first thing comes to
mind is bubble sort. So the problem is sorting and solution is bubble sort. Same
holds true for design patterns.
Which are the three main
categories of design patterns?
There are three basic classifications of patterns
Creational, Structural, and Behavioral patterns.
Creational Patterns
·
Abstract
Factory:-
Creates an
instance of several families of classes
·
Builder:
- Separates object
construction from its representation
·
Factory
Method:- Creates an instance of
several derived classes
·
Prototype:- A fully initialized
instance to be copied or cloned
·
Singleton:- A class in which only a
single instance can exist
Note:
- The best way
to remember Creational pattern is by ABFPS (Abraham Became First President of
States).
Structural Patterns
·
Adapter:-Match interfaces of
different classes.
·
Bridge:-Separates an object’s
abstraction from its implementation.
·
Composite:-A tree structure of simple
and composite objects.
·
Decorator:-Add responsibilities to
objects dynamically.
·
Façade:-A single class that
represents an entire subsystem.
·
Flyweight:-A fine-grained instance
used for efficient sharing.
·
Proxy:-An object representing another object.
Note :
To remember
structural pattern best is (ABCDFFP)
Behavioral Patterns
· Mediator:-Defines simplified
communication between classes.
· Memento:-Capture and restore an
object's internal state.
· Interpreter:- A way to include language
elements in a program.
·
Iterator:-Sequentially access the
elements of a collection.
· Chain of Resp:
- A way of passing a request
between a chain of objects.
· Command:-Encapsulate a command
request as an object.
· State:-Alter an object's behavior when its state changes.
· Strategy:-Encapsulates an algorithm
inside a class.
· Observer:
- A way of notifying change
to a number of classes.
· Template
Method:-Defer the exact steps of an algorithm to a subclass.
· Visitor:-Defines a new operation to
a class without change.
Note: - Just remember
Music....... 2 MICS On TV (MMIICCSSOTV).
Note :- In the further section we
will be covering all the above design patterns in a more detail
manner.
Can you explain factory
pattern?
Factory pattern is one of the types of creational
patterns. You can make out from the name factory itself it’s meant to construct
and create something. In software architecture world factory pattern is meant to
centralize creation of objects. Below is a code snippet of a client which has
different types of invoices. These invoices are created depending on the invoice
type specified by the client. There are two issues with the code below:-
Ø
First we have lots of ‘new’ keyword scattered in the client. In
other ways the client is loaded with lot of object creational activities which
can make the client logic very complicated.
Ø
Second issue is that the client needs to be aware of all types of
invoices. So if we are adding one more invoice class type called as
‘InvoiceWithFooter’ we need to reference the new class in the client and
recompile the client also.
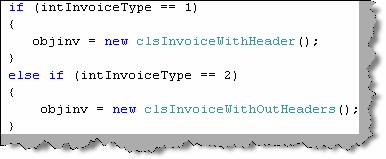
Figure: -
Different types of invoice
Taking these issues as our base we will now look in to
how factory pattern can help us solve the same. Below figure ‘Factory Pattern’
shows two concrete classes ‘ClsInvoiceWithHeader’ and
‘ClsInvoiceWithOutHeader’.
The first issue was that these classes are in
direct contact with client which leads to lot of ‘new’ keyword scattered in the
client code. This is removed by introducing a new class ‘ClsFactoryInvoice’
which does all the creation of objects.
The second issue was that the client code is
aware of both the concrete classes i.e. ‘ClsInvoiceWithHeader’ and
‘ClsInvoiceWithOutHeader’. This leads to recompiling of the client code when we
add new invoice types. For instance if we add ‘ClsInvoiceWithFooter’ client code
needs to be changed and recompiled accordingly. To remove this issue we have
introduced a common interface ‘IInvoice’. Both the concrete classes
‘ClsInvoiceWithHeader’ and ‘ClsInvoiceWithOutHeader’ inherit and implement the
‘IInvoice’ interface.
The client references only the ‘IInvoice’ interface
which results in zero connection between client and the concrete classes (
‘ClsInvoiceWithHeader’ and ‘ClsInvoiceWithOutHeader’). So now if we add new
concrete invoice class we do not need to change any thing at the client side.
In one line the creation of objects is taken care by
‘ClsFactoryInvoice’ and the client disconnection from the concrete classes is
taken care by ‘IInvoice’ interface.
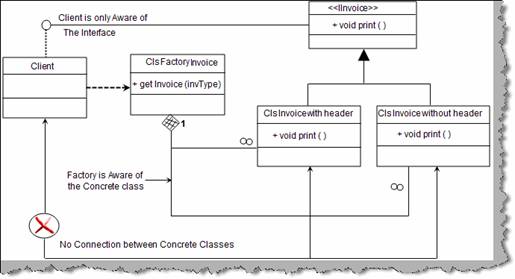
Figure: -
Factory pattern
Below are the code snippets of how actually factory
pattern can be implemented in C#. In order to avoid recompiling the client we
have introduced the invoice interface ‘IInvoice’. Both the concrete classes
‘ClsInvoiceWithOutHeaders’ and ‘ClsInvoiceWithHeader’ inherit and implement the
‘IInvoice’ interface.
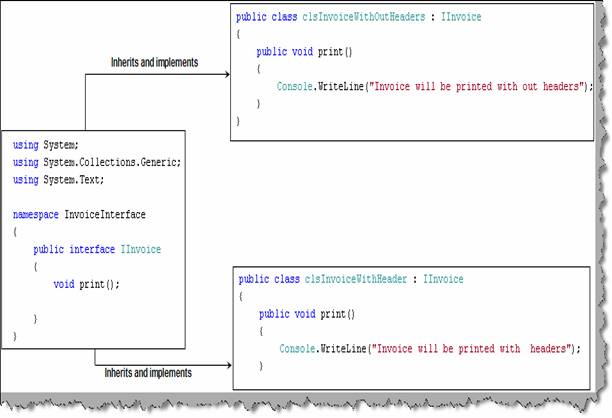
Figure :-
Interface and concrete classes
We have also introduced an extra class
‘ClsFactoryInvoice’ with a function ‘getInvoice()’ which will generate objects
of both the invoices depending on ‘intInvoiceType’ value. In short we have
centralized the logic of object creation in the ‘ClsFactoryInvoice’. The client
calls the ‘getInvoice’ function to generate the invoice classes. One of the most
important points to be noted is that client only refers to ‘IInvoice’ type and
the factory class ‘ClsFactoryInvoice’ also gives the same type of reference.
This helps the client to be complete detached from the concrete classes, so now
when we add new classes and invoice types we do not need to recompile the
client.
|