|
Technologies
|
|
|
|
|
|
|
|
|
|
Data Encryption and Decryption using DPAPI classes in .NET
|
|
|
By
Balamurali Balaji
Posted On
Apr 16,2008
|
Article Rating:
No of Comments:
9
|
Category:
.Netframework
|
|
Data Encryption and Decryption using
DPAPI classes in .NET
This article explains how to use the
ProtectMemory and ProtectData classes in System.Security.Cryptography namespace
to encrypt and decrypt data by calling wrappers around DPAPI methods.
Introduction
Data Protection API (DPAPI) is a
security encryption module introduced in Windows 2000 and is included with the
later versions of Windows to provide cryptographic features like key management,
to secure user credentials. This is a service that is
provided by the operating system and does not require additional libraries. It
provides encryption for sensitive data in memory.
|
|
DPAPI generates two
keys: a key for the user credential, and a master key. It also uses random
session key when you call CryptProtectData. Combine with all these keys, data is
protected. When you change the password, DPAPI will hook up to the password
change event and does the re-encryption whole again. DPAPI works at both the
machine-level and user-level encryption access scenarios.
In .NET 2.0 and later versions, a set
of wrapper classes have been introduced to encrypt data by simple means using
the current user account or computer accessing DPAPI. You need not have to use
the P/Invoke to work with the DPAPI methods and the encryption-decryption is not
just limited to user credentials.
ProtectedMemory
The ProtectedMemory class may be used
to encrypt an array of in-memory bytes. This functionality is available in
Microsoft Windows XP and later operating systems. You can specify that memory
encrypted by the current process can be decrypted by the current process only,
by all processes, or from the same user context. The MemoryProtectionScope
enumeration is available for this purpose.
To perform encryption and decryption of
data stored in-memory, you may use the methods Protect and UnProtect
methods.
Example:
To demonstrate this, create a new
console application and add reference to System.Security.dll. In the program.cs,
add the following code:
static void Main(string[]
args)
{
byte[] myData = new
byte[32];
System.Text.ASCIIEncoding ae =
new ASCIIEncoding();
// Protecting and
Un-protecting data in memory
Console.Write("Enter some
text: ");
string text =
Console.ReadLine();
if (text.Length <
myData.Length)
text =
text.PadRight(myData.Length, ' ');
else
text =
text.Substring(0,myData.Length);
myData =
ae.GetBytes(text);
Console.WriteLine("Before
protection: {0}", ae.GetString(myData));
System.Security.Cryptography.ProtectedMemory.Protect(myData,
System.Security.Cryptography.MemoryProtectionScope.SameProcess);
Console.WriteLine("After
protection: {0}", ae.GetString(myData));
System.Security.Cryptography.ProtectedMemory.Unprotect(myData,
System.Security.Cryptography.MemoryProtectionScope.SameProcess);
Console.WriteLine("After
un-protection: {0}", ae.GetString(myData));
}
|
|
|
Both the Protect and UnProtect methods
takes the input data only in multiples of 16 bytes. In the above program, the
maximum length of the data to be encrypted in 32 bytes; if the user enters data
more or less, the entered text is trimmed and padded with white spaces
accordingly. Below is the output displaying both the encrypted and decrypted
data you entered on the console.
Output:
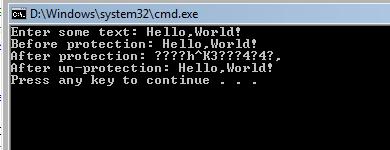
ProtectedData
The ProtectedData class provides access
to the Data Protection API (DPAPI) available in Microsoft Windows 2000 and later
operating systems. This is a service that is provided by the operating system
and does not require additional libraries. It provides protection using the user
or machine credentials to protect or unprotect data.
The class consists of two wrappers for
the unmanaged DPAPI, Protect and Unprotect. These two methods can be used to
protect and unprotect data such as passwords, keys, and connection
strings.
Example:
To demonstrate the usage of
ProtectedData class, in the Main method, add the following code:
byte[] myEntropy = { 1, 2, 3,
4, 5, 6 };
byte[] protectedData =
System.Security.Cryptography.ProtectedData.Protect(myData, myEntropy,
System.Security.Cryptography.DataProtectionScope.CurrentUser);
Console.WriteLine("After data
protection: {0}", ae.GetString(protectedData));
byte[] unprotectedData =
System.Security.Cryptography.ProtectedData.Unprotect(protectedData, myEntropy,
System.Security.Cryptography.DataProtectionScope.CurrentUser);
Console.WriteLine("After
un-protection: {0}", ae.GetString(unprotectedData));
In the above code, both the Protect and
UnProtect methods use the optionalEntropy parameter that provides additional
information stored as a byte array to encrypt and decrypt data. This would give
more protection over your data.
Output:
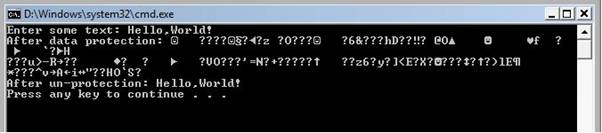
|
|
Summary:
The ProtectMemory and ProtectData
classes comes in handy for encrypting and decrypting any data on the fly and you
need not have to rely on any algorithms. It uses the Windows Operating System’s
DPAPI security module and can be implemented across the applications, users and
machines. Its real potential lays in its application in the distributed
computing which is not the scope of the article.
|
|
Similar Articles
You can contribute to CodeDiget.Com:
|
Article Feedback
|
Comments
|
OK5mF9 , [url=http://hcfacynfuioy.com/]hcfacynfuioy[/url], [link=http://xjrvezgerjem.com/]xjrvezgerjem[/link], http://poaxsgtsjirp.com/
OK5mF9 , [url=http://hcfacynfuioy.com/]hcfacynfuioy[/url], [link=http://xjrvezgerjem.com/]xjrvezgerjem[/link], http://poaxsgtsjirp.com/
Commented By
OK5mF9 , [url=http://hcfacynfuioy.com/]hcfacynfuioy[/url], [link=http://xjrvezgerjem.com/]xjrvezgerj
on
3/23/2012 @ 12:54 PM
|
rQlSMB <a href="http://mdnjfedyfpzd.com/">mdnjfedyfpzd</a>
rQlSMB <a href="http://mdnjfedyfpzd.com/">mdnjfedyfpzd</a>
Commented By
rQlSMB <a href="http://mdnjfedyfpzd.com/">mdnjfedyfpzd</a>
on
3/22/2012 @ 5:56 PM
|
SQvnqc , [url=http://jxhvjodzdzmk.com/]jxhvjodzdzmk[/url], [link=http://pnljkdqgevge.com/]pnljkdqgevge[/link], http://ftesxaffpmeo.com/
SQvnqc , [url=http://jxhvjodzdzmk.com/]jxhvjodzdzmk[/url], [link=http://pnljkdqgevge.com/]pnljkdqgevge[/link], http://ftesxaffpmeo.com/
Commented By
SQvnqc , [url=http://jxhvjodzdzmk.com/]jxhvjodzdzmk[/url], [link=http://pnljkdqgevge.com/]pnljkdqgev
on
3/21/2012 @ 9:57 PM
|
puUAxc <a href="http://iwadqqqedpdz.com/">iwadqqqedpdz</a>
puUAxc <a href="http://iwadqqqedpdz.com/">iwadqqqedpdz</a>
Commented By
puUAxc <a href="http://iwadqqqedpdz.com/">iwadqqqedpdz</a>
on
3/21/2012 @ 5:09 PM
|
1. Transposition ciphers can be seplmir, allowing the sender and receiver to communicate with a minimum of effort exhausted learning and employing the shift or permutation of the plaintext being used.
1. Transposition ciphers can be seplmir, allowing the sender and receiver to communicate with a minimum of effort exhausted learning and employing the shift or permutation of the plaintext being used. While someone using a substitution cipher would have to look up each individual symbol and rewrite the message, someone reading a transposition cipher would just have to memorize the key, then shift or rearrange the ciphered message to reveal the intended message. The disadvantage of this type of cipher is that it is not very secure. Once someone figures out the key or shift, they can then decipher any message using that particular key. In substitution ciphers, they must figure out the meaning of each different symbol, with no correlation to each other. 2. People may need to use a cipher or code to keep a variety of things secret. For example, if a husband was meeting with a group of friends to go out gambling once a week, but his wife prohibited him from engaging in such activity, he and his friends could communicate in code. Alternatively, email is a very important means of communication that requires encoding to protect people's security and private information. If anyone were able to intercept and read everyone's emails, people would stop using emails to communicate with each other. This would apply to almost all other means of internet-driven communication. 3. Well, first off, our culture and society is far more advanced than the early Muslim civilization. The way we educate our children significantly impacts the way they think and look at the world. We are taught to pay attention to spelling, numbers, and facts. Naturally many people would focus on the letters or symbols that recur most frequently. Then a natural progression would be to compare those recurrences to a sample of pain text. 4.Singh means that people rely on codes and ciphers too much. When they believe their code is unbreakable, they write whatever they want, no matter how incriminating it may be, since they are confident that no one will be able to interpret the message, even if it is intercepted. When not using a code or cipher, people trying to keep a secret will be more carful in what they write down, and try not to give anything away or provide valuable information for those who may intercept the message. If this same level of precaution were taken in conjunction with a code or cipher, evidence would be extremely difficult to find.
Commented By
1. Transposition ciphers can be seplmir, allowing the sender and receiver to communicate with a mini
on
3/21/2012 @ 9:08 AM
|
Colin Forbes
xnfz02sshu7eglm9
<a href= http://ycohlwqvzugq.com >kyghnga liowhrt</a> http://qvdnen.com <a href= http://tdrobqf.com >rages msmk</a> http://jtudwhpx.com <a href= http://izmcwt.com >ytejrr rsrl</a> http://gfunkrn.com <a href= http://bhyozubchpa.com >awilds fdugryb</a> http://vsmkrrymyt.com
Commented By
Mark Berger
on
10/20/2008 @ 12:38 AM
|
DPAPI on the network
I need the ability to protect and un-protect a connection string in my config file on a Windows Forms application using VS2005. I am using Click Once Deployment to a server from which a user will install the applicaiton on their PC. From everything I have read, this cannot be done using DPAPI. Am I correct? If I am not correct, then how can it be done? If I am correct, what method of encryption should I use?
Commented By
Greyhound
on
7/23/2008 @ 11:43 AM
|
Mr
Hi,
DPAPI works at both the machine and user level. I have mentioned this in the Introduction section. For example, In a networked environment, once you protect a password or config file specifying the scope as machine or user, un-protection would effect only at the particular machine and user. Otherwise, encryption and decryption must be available to all the machines/users especially when you have a option of storing the protected data in a file.
I have not illustrated this in this article, as I felt to write about the simple use of ProtectMemory and ProtectData classes and I do not have a network to test the same.
-Balamurali Balaji
Commented By
Balamurali Balaji
on
4/19/2008 @ 6:55 AM
|
Not portable
You should mention that data encrypted on one machine and decrypted on another will not work. This only works on a single machine and is meant for encrypting/decrypting the config file to hide a password.
Commented By
pb
on
4/19/2008 @ 5:16 AM
|
|
|
|
|
|
|
|
|
|