Steps
1. Create a new Asp.Net project in your Visual Studio 2008 with the language of your choice.
2. Download the new jQuery library from jQuery.com and integrate it
into your Asp.Net project. The following Faq’s will help you to integrate jQuery library into
your project.
What is jQuery? How to use it in ASP.Net
Pages?
How to enable jQuery intellisense in Visual Studio
2008?
How to use jQuery intellisense in an external
JavaScript file?
3. Download the latest version of jCrop from jCrop official site. The current version as
of this writing is 0.9.8
4. Unzip the file jquery.Jcrop-0.9.8.zip. Copy jquery.Jcrop.min.js file
from Jcrop\js and jquery.Jcrop.css, Jcrop.GIF from Jcrop\css to a
folder called _script in your project solution.
5. Assuming you have integrated jQuery library, let’s move forward and
use the jCrop plug-in to crop an image. For easy understanding, I have copied an
image called “Cartoons.jpg” in a folder called Images in our project solution.
We will use this image to understand how cropping works using Jcrop plug-in. The
solution explore will look like below,
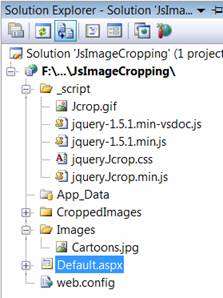
How Image Cropping works in
jCrop?
The jCrop plug-in allows the user to
select a part of image from a larger image using mouse cursor. The selected part
can then moved or changed accordingly to fit our need. Something like
below,
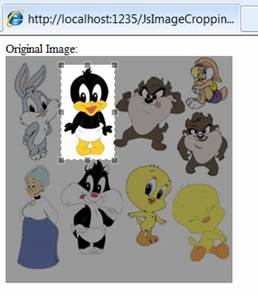
Once the cropping area is selected like
above, the jCrop plug-in will return x,y co-ordinate value which indicates the
start of the selection with height and width parameter values. The Jcrop plug-in
exposes OnSelect/OnChange event which allows us to capture these values after
the selection. The captured values can be sent to the server where we can slice
the selected part of the original image using Graphics class in System.Drawing
namespace. In our example, the final output will be something like
below,
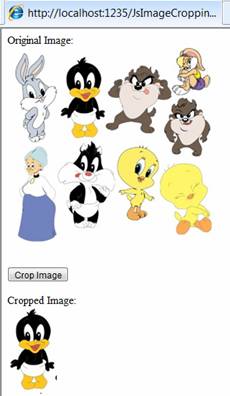
To enable Jcrop on an image, we can use
the below code,
$('#ImFullImage').Jcrop();
In our example, we can capture x,y
co-ordinates value, length and width to hidden fields which can be accessed in
the code behind file to crop from the original image. To do this, drag 4
HiddenField controls(to capture x,y, height, width), 2 Image controls(1 for
original image and another for cropped image) and a Button control to do the
crop in your Default.aspx page. On click of the Button, we can crop the image
and save it to a folder called CroppedImages using Graphics API in
System.Drawing namespace. The cropped image can be finally displayed in the
Image control as seen in the above figure.
The final code will be,
Default.aspx
<script
src="_script/jquery-1.5.1.min.js"
type="text/javascript"></script>
<script
src="_script/jquery.Jcrop.min.js"
type="text/javascript"></script>
<link
href="_script/jquery.Jcrop.css" rel="stylesheet" type="text/css"
/>
<script
type="text/javascript">
$(function() {
$('#ImFullImage').Jcrop({
onSelect:
updateCoords,
onChange:
updateCoords
});
});
function updateCoords(c)
{
$('#hfX').val(c.x);
$('#hfY').val(c.y);
$('#hfHeight').val(c.h);
$('#hfWidth').val(c.w);
}
</script>
</head>
<body>
<form id="form1"
runat="server">
<asp:HiddenField ID="hfX"
runat="server" />
<asp:HiddenField ID="hfY"
runat="server" />
<asp:HiddenField ID="hfHeight"
runat="server" />
<asp:HiddenField ID="hfWidth"
runat="server" />
<div>
Original Image: <br
/>
<asp:Image ID="ImFullImage"
ImageUrl="~/Images/Cartoons.jpg" runat="server" /><br />
<asp:Button ID="btnCrop"
runat="server" Text="Crop Image"
onclick="btnCrop_Click"
/><br />
</div>
<br />
<div>
Cropped Image:<br
/>
<asp:Image ID="imCropped"
runat="server" />
</div>
</form>
</body>
</html>
|