What is QueryExtender control?
A QueryExtender control can be used to
do efficient filtering on data at the database end with the help of LINQ
technology. This means this control works adjacent with the LINQDataSource and
EntityDataSource control to do
filteration at the back end of your application.
Pre-Requisites
Visual
Studio 2010 and ASP.Net 4.0
The current version of visual studio
2010 and .Netframework 4.0 is beta 1. You can download the Visual Studio 2010
beta 1 and .net FX 4 from here.
To know more about downloading and installing newer version of Visual Studio,
please refer here. Download
the products and install it.
Note
Since the products are still in
beta, the functionality discussed here are subject to change. If you have
feedback or bug, please submit at the visual studio
support page.
To have a better understanding, we will
build a sample application that searches employee information from the table
Employees.
Steps
1. Open Visual Studio 2010.
2. Create a new Asp.Net Website.
3. From the solution explorer, add a new SQL express database inside App_Data folder and create
a table called Employees.
Refer my Server explorer,
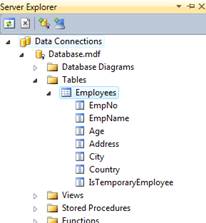
As i said earlier, the new
QueryExtender control will work along with LINQDataSource control. Hence, to
proceed with we need to first design the LINQ to SQL classes.
To do this, right click your project in
solution explorer and click “Add New Item..”. Select “Linq to SQL Classes” and
click OK. You can rename the class if you require.
Creating the LINQ to SQL
classes
Open Server Explorer, drag the Employee table into LINQ to SQL
designer and click Save to create the LINQ to SQL classes.
Refer the below figure.
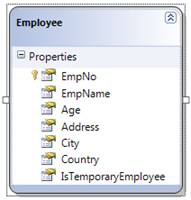
Next, we will design our search page
with the help of LINQDataSource control and QueryExtender control.
Creating Search Page
Drag a GridView control and
LINQDataSource control from the data tab of visual studio 2010. Configure the
LINQDataSource control to use our DataContext class. Next, configure the
GridView control’s DataSourceID property to the ID of LINQDataSource control.
Refer the code below,
<asp:GridView ID="GridView1"
runat="server" AutoGenerateColumns="False"
DataKeyNames="EmpNo"
DataSourceID="LinqDataSource1">
<Columns>
<asp:BoundField
DataField="EmpNo" HeaderText="EmpNo" InsertVisible="False"
ReadOnly="True"
SortExpression="EmpNo" />
<asp:BoundField
DataField="EmpName" HeaderText="EmpName"
SortExpression="EmpName"
/>
<asp:BoundField
DataField="Age" HeaderText="Age"
SortExpression="Age"
/>
<asp:BoundField
DataField="Address" HeaderText="Address"
SortExpression="Address"
/>
<asp:BoundField
DataField="City" HeaderText="City" SortExpression="City" />
<asp:BoundField
DataField="Country" HeaderText="Country"
SortExpression="Country"
/>
<asp:CheckBoxField
DataField="IsTemporaryEmployee"
HeaderText="IsTemporaryEmployee" SortExpression="IsTemporaryEmployee"
/>
</Columns>
</asp:GridView>
<asp:LinqDataSource
ID="LinqDataSource1" runat="server"
ContextTypeName="DataClassesDataContext" EntityTypeName=""
TableName="Employees">
</asp:LinqDataSource>
Execute the page. You can see the
employee information populated on the screen. Next, we will add some search
functionality in the page and use QueryExtender control to configure
search.
To have a simple understanding, we
build our search page that searches based on the employee name typed in a
textbox control. To do this, we will drag a TextBox control and Button control
to the page.
Now, we will add a QueryExtender
control that uses our LINQDataSource control to provide search based on
employee name. The QueryExtender control will perform the filtration based on
the filtration parameter configured with the QueryExtender control. In our case,
it is SearchExpression that needs to be configured to get the parameter value
from textbox control for searching.
Refer the code below,
Name: <asp:TextBox ID="txtName"
runat="server"></asp:TextBox><br />
<asp:Button ID="Button1" runat="server"
Text="Search" />
//GridView code, Refer the code in
previous section
<asp:LinqDataSource
ID="LinqDataSource1" runat="server"
ContextTypeName="DataClassesDataContext" EntityTypeName=""
TableName="Employees">
</asp:LinqDataSource>
<asp:QueryExtender
ID="QueryExtender1" TargetControlID="LinqDataSource1"
runat="server">
<asp:SearchExpression
DataFields="EmpName"
SearchType="StartsWith">
<asp:ControlParameter
ControlID="txtName" />
</asp:SearchExpression>
</asp:QueryExtender>
When you execute the page, you may get
the following error “Unknown server tag 'asp:SearchExpression'” with
visual Studio 2010 beta 1.
To fix this error, add the following
tag prefix and namespace to the controls section of Pages tag in web.config
file,
<pages>
<controls>
<add tagPrefix="asp"
namespace="System.Web.UI.WebControls.Expressions"
assembly="System.Web.Extensions, Version=4.0.0.0, Culture=neutral,
PublicKeyToken=31BF3856AD364E35" />
....
</controls>
</pages>
Now, execute the page and you can see
the search in section.
Refer the below figure,
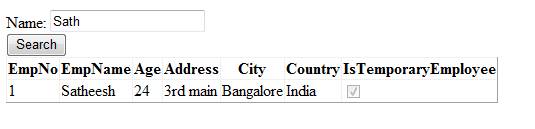
In coming sections, we will try to
implement more searching options the QueryExtender offers us.
|